How to Bash Ranges
-
Use the
for
Loop to Get Range in Bash -
Use the
for
Loop With Three-Expression to Get Range in Bash -
Use the
while
Loop to Get Range in Bash -
Use
eval
to Get Range in Bash -
Use
seq
to Get Range in Bash
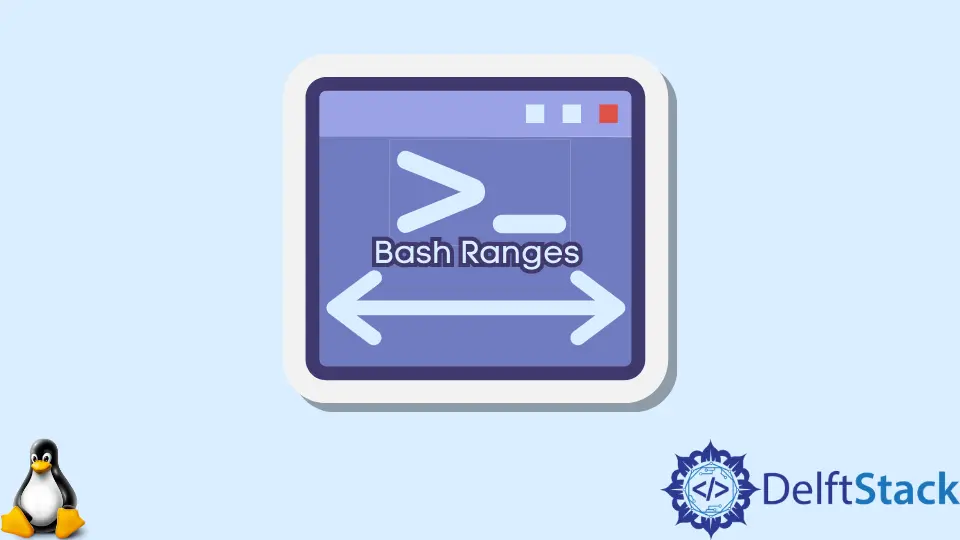
This tutorial demonstrates iterating through a range of integers in bash with for
loop and while
loop.
Use the for
Loop to Get Range in Bash
The bash script below uses a for
loop with brace expansion to loop through a range of numbers. The numbers inside the braces are incremented sequentially.
#!/bin/bash
printf "Print Numbers from 0 to 5\n"
for x in {0..5}
do
printf "number:$x\n"
done
Output:
Print Numbers from 0 to 5
number:0
number:1
number:2
number:3
number:4
number:5
Use the for
Loop With Three-Expression to Get Range in Bash
The script uses a for
loop with a three-expression like in C language. Here, expr1
is the initialization, expr2
is the condition, and expr3
is the increment.
In our case, x
is initialized to 0
, x
is tested if it is less than or equal to 5
, and lastly, x
is incremented by 1
.
#!/bin/bash
max=5
printf "Print Numbers from 0 to $max\n"
for ((x=0;x<=max;x++)); do
printf "number: $x\n"
done
Output:
Print Numbers from 0 to 5
number: 0
number: 1
number: 2
number: 3
number: 4
number: 5
Use the while
Loop to Get Range in Bash
This uses a while
loop with a binary comparison operator, -le
, that is used for arithmetic value comparison.
In our case, the while loop executes as long as x
is less than or equal to the variable $max
.
#!/bin/bash
x=0
max=5
printf "Print numbers from $x to $max\n"
while [ $x -le $max ]; do
printf "number: $x\n"
x=$(($x+1))
done
Output:
Print numbers from 0 to 5
number: 0
number: 1
number: 2
number: 3
number: 4
number: 5
Use eval
to Get Range in Bash
eval
is a bash command used to execute arguments as shell commands. In the script below, the braces generate numbers from 0
to $max
in increments of 1
, the for
loop iterates over these numbers, and the printf
command displays them.
#!/bin/bash
max=4
printf "Print Numbers from 0 to $max\n"
for x in `eval echo {0..$max}`
do
printf "number: $x\n"
done
Output:
Print Numbers from 0 to 4
number: 0
number: 1
number: 2
number: 3
number: 4
Use seq
to Get Range in Bash
seq
is a bash command used to generate numbers from start
to finish
in increment steps.
In our case, seq
generates numbers from x
to $max
in increments of 1
. The for
loop iterates over these numbers, and the printf
command is used to display them.
x=0
max=5
printf "Print numbers from $x to $max\n"
for x in $(seq $x $max)
do
printf "number: $x\n"
done
Output:
Print numbers from 0 to 5
number: 0
number: 1
number: 2
number: 3
number: 4
number: 5