How to Get User Input in Linux Shell Script
Yahya Irmak
Feb 02, 2024
-
Use
read
to Get Input in Linux -
Use
select
to Give Options in Linux -
Use
whiptail
to Get Input via GUI in Linux
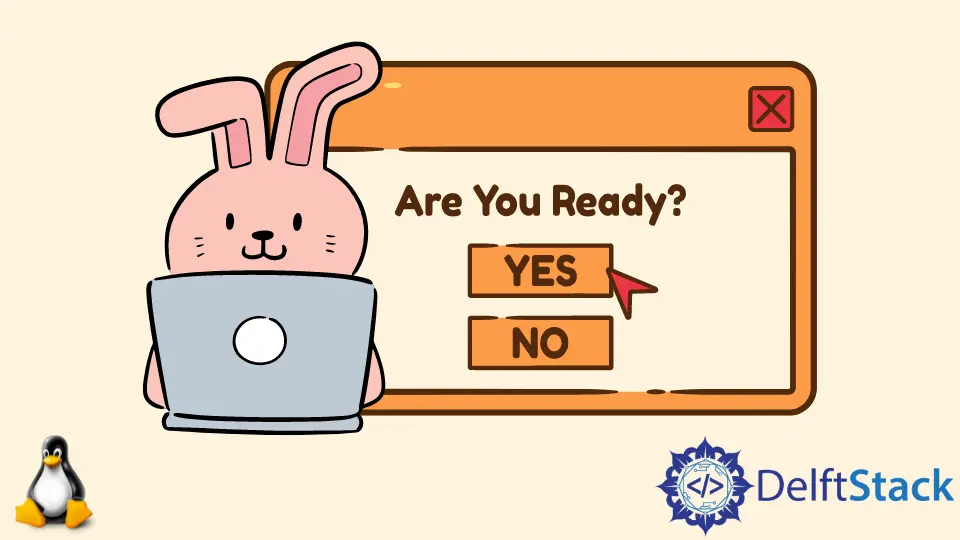
This article will explain how to get user input in Linux Shell Script and give some examples.
read
: to receive input from the userselect
: to provide optionswhiptail
: to use the user interface
Use read
to Get Input in Linux
The read
command takes an input from the user and stores it in a variable.
read -p "Do you want to continue? (Y/y for Yes, any other key for No) " answer
case $answer in
[Yy]* ) echo "Program continues..."; break;;
* ) echo "Program exits."; exit;;
esac
Output:
Use select
to Give Options in Linux
The select
command gives options to the user and stores the answer in a variable.
echo "Do you want to continue? "
select answer in "Yes" "No"; do
case $answer in
Yes ) echo "Program continues..."; break;;
No ) echo "Program exits."; exit;;
esac
done
Use whiptail
to Get Input via GUI in Linux
The whiptail
command provides Yes/No
options in the visual interface.
dialog
, gdialog
, or kdialog
can be used depending on the system.
if whiptail --yesno "Do you want to continue? " 10 40 ;then
echo "Program continues...";
else
echo "Program exits."
fi
Author: Yahya Irmak
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn