How to Take Optional Input Arguments in Bash
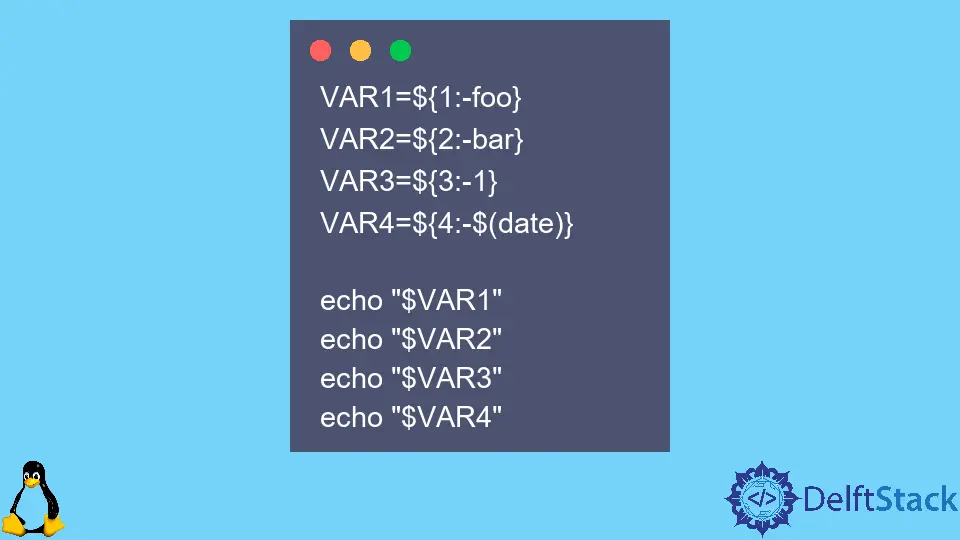
Sometimes we need to create a dynamic function that can execute in both without passing any argument or passing arguments. To do this, we need to set some default values for these arguments so that if the arguments didn’t pass when calling the function, it could use the default arguments.
This article will demonstrate how to create optional input arguments for a function. We will also look at some examples and explanations to make the topic easier.
Take Optional Input Arguments in Bash
In the below example, we will be printing the default values. We declared 4 variables that contain default values.
The code for our example will look like the following:
VAR1=${1:-foo}
VAR2=${2:-bar}
VAR3=${3:-1}
VAR4=${4:-$(date)}
echo "$VAR1"
echo "$VAR2"
echo "$VAR3"
echo "$VAR4"
After code execution, we will get the below output:
foo
bar
1
Sat Aug 13 14:59:31 +06 2022
Now let’s see an advanced example. In the example shared below, we created a function that can work with 0, 1, and 2 parameters and set a default value inside the function.
Now, the code for our example looks like the following:
FindSum()
{
VAR1=${1:-40}
VAR2=${2:-50}
if [ $# -lt 1 ];
then
echo "The provided parameters are: $VAR1, $VAR2."
elif [ $# -lt 2 ];
then
echo
echo "The provided parameters is: $VAR2."
else
echo
echo "No parameters provided"
VAR1=$1
VAR2=$2
fi
SUM=$((VAR1+VAR2))
echo "The sum of $VAR1 and $VAR2 is $SUM"
}
FindSum
FindSum 40
FindSum 70 30
Let’s explain the code part by part.
First, we set the variables’ default values using the lines VAR1=${1:-40}
and VAR2=${2:-50}
. After that, we checked whether or not the value for these variables was given.
If the value doesn’t provide them, we have to use its default value; otherwise, use the value given by the user.
Lastly, we just performed a sum operation using the line SUM=$((VAR1+VAR2))
and printed the result. Here, we called the function without any argument by FindSum
.
Again, we called the function with one argument using the line FindSum 40
. And we called the function with two arguments using the line FindSum 70 30
.
The provided parameters are: 40, 50.
The sum of 40 and 50 is 90
The provided parameters is: 50.
The sum of 40 and 50 is 90
No parameters provided
The sum of 70 and 30 is 100
All the codes used in this article are written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn