Single Line if...else in Bash
- What is a Single Line if…else in Bash?
- Example of Single Line if…else
- Using Single Line if…else with Git Commands
- Advantages of Single Line if…else Statements
- Best Practices for Using Single Line if…else Statements
- Conclusion
- FAQ
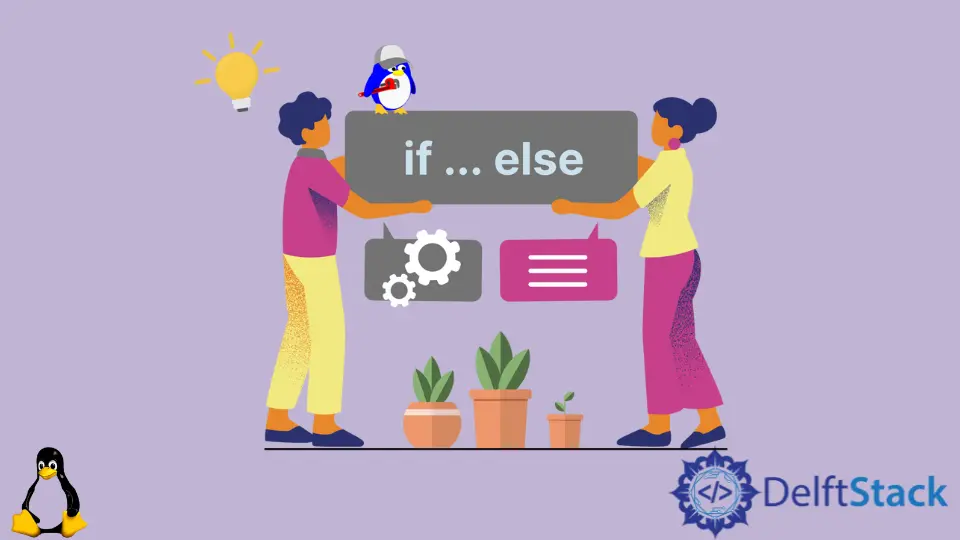
When it comes to scripting in Bash, efficiency and brevity are often key. One of the most effective ways to streamline your scripts is through the use of single line if…else statements.
This tutorial will discuss the single line if-else in Bash, showing you how to implement this feature effectively. Whether you’re a seasoned developer or just starting with Bash scripting, understanding how to use single line if-else statements can save you time and enhance the readability of your code.
In this article, we will cover various methods to implement this, along with practical examples and explanations to ensure you grasp the concepts fully.
What is a Single Line if…else in Bash?
A single line if…else statement in Bash allows you to execute conditional commands without breaking your code into multiple lines. This is particularly useful for simple conditions where a full block of code would be unnecessarily verbose. The syntax is straightforward and can help keep your scripts clean and efficient.
The basic syntax for a single line if…else statement looks like this:
if condition; then command1; else command2; fi
This structure allows you to check a condition and execute one command if the condition is true and another if it is false, all in one line.
Example of Single Line if…else
Let’s take a look at a practical example using a single line if…else statement in Bash. Suppose you want to check if a file exists in your current directory and print a message based on that.
[ -f myfile.txt ] && echo "File exists" || echo "File does not exist"
Output:
File exists
In this example, [ -f myfile.txt ]
checks if the file myfile.txt
exists. If it does, echo "File exists"
will execute. If the file does not exist, the command after ||
executes, printing “File does not exist”. This is a compact way to handle conditional logic in your scripts.
Using Single Line if…else with Git Commands
If you’re working with Git, you can also apply single line if…else statements to streamline your version control tasks. For example, you may want to check if your current branch is up to date with the remote repository.
git fetch origin && git status | grep 'up to date' && echo "Branch is up to date" || echo "Branch is not up to date"
Output:
Branch is up to date
In this command, git fetch origin
updates your local copy of the repository. The git status
command checks the status of your current branch. If the output contains the phrase “up to date”, the script will print “Branch is up to date”. If not, it will print “Branch is not up to date”. This method allows you to quickly assess your Git status without excessive commands.
Advantages of Single Line if…else Statements
Single line if…else statements provide several advantages in Bash scripting. First, they enhance readability by reducing the amount of code you need to write. This is particularly beneficial for simple conditions where a multi-line block would be overkill. Second, they can help you maintain a cleaner script, making it easier to follow and debug.
Moreover, using single line statements can improve the efficiency of your scripts. By condensing your logic into fewer lines, you minimize the overhead associated with creating and managing multiple code blocks. This can lead to faster execution times, especially in scripts that require frequent conditional checks.
Best Practices for Using Single Line if…else Statements
While single line if…else statements can be powerful, it’s essential to use them judiciously. Here are some best practices to keep in mind:
- Keep it Simple: Use single line statements for straightforward conditions. If your logic becomes complex, consider breaking it into multiple lines for clarity.
- Use Clear Conditions: Ensure that the conditions you are checking are easy to understand. This will help others (and your future self) quickly grasp the logic behind your script.
- Test Thoroughly: Always test your single line statements to confirm they behave as expected. This is especially important when dealing with critical operations like Git commands.
By following these best practices, you can maximize the effectiveness of single line if…else statements in your Bash scripts.
Conclusion
Single line if…else statements in Bash provide a powerful way to simplify your scripts, particularly when working with Git commands. By understanding how to implement these statements effectively, you can enhance the readability and efficiency of your code. Whether you’re checking file existence or assessing your Git branch status, mastering this technique will undoubtedly make your scripting tasks easier and more enjoyable. So, next time you write a Bash script, consider using single line if…else statements to streamline your code!
FAQ
-
What is a single line if…else statement in Bash?
A single line if…else statement allows you to execute conditional commands in a compact format, improving script readability. -
How do I check if a file exists using a single line if…else in Bash?
You can use the syntax[ -f filename ] && echo "File exists" || echo "File does not exist"
. -
Can I use single line if…else with Git commands?
Yes, you can streamline Git commands using single line if…else statements for quick checks. -
What are the advantages of using single line if…else statements?
They enhance readability, reduce code length, and improve efficiency for simple conditions. -
Are there any best practices for using single line if…else statements?
Keep conditions simple, ensure clarity, and thoroughly test your statements to confirm they work as intended.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn