The -ne Operator in Bash
-
Compare Strings Using the Not Equal Operator
-ne
in Bash -
Compare Numbers Using the Not Equal Operator
-ne
in Bash
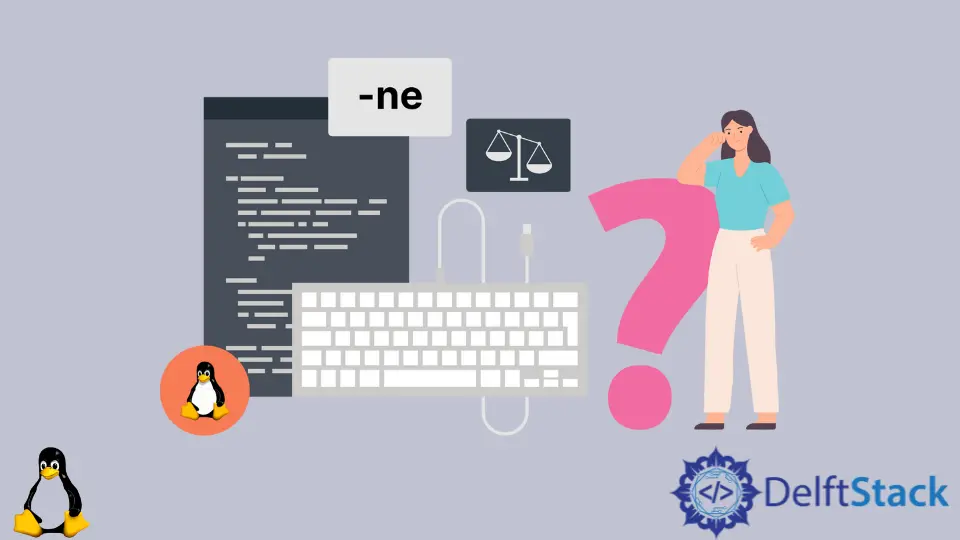
If two potential values are not equal, the -ne
operator is used in Bash programming to compare them. In Bash, the not equal
function is represented by the -ne
character.
The !=
operator is used to express inequality. The logical outcome of the operation not equal
is True
or False
.
The not equal
expression is frequently combined with if
or elif
expressions to test for equality and execute sentences. -ne
only works when brackets surround it [[]]
.
[[Value1 -ne Value2]]
Value1
is generally a bash variable compared toValue2
, which is a number.-ne
cannot be used with the string types; instead, it throws an exception in the terminal that saysinteger expression expected
.!=
is used to compare strings.
Compare Strings Using the Not Equal Operator -ne
in Bash
As mentioned, we will use !=
to compare the strings. Let’s look at an example.
#!/bin/bash
nameone="Bobby"
nametwo="Shera"
if [[ $nameone != $nametwo ]]; then
echo "Not Equal!"
else
echo "Equal!"
fi
We declared two string variables, nameone
with the value Bobby
and nametwo
with the value Shera
, and compared them using !=
.
Output:
Not Equal!
Compare Numbers Using the Not Equal Operator -ne
in Bash
We will use -ne
to compare numbers. We will declare two integer variables, numone
with the value 8
and numtwo
with the value 9
and compare them using -ne
.
#!/bin/bash
numone=8
numtwo=9
if [[ $numone -ne $numtwo ]]; then
echo "Not Equal!"
else
echo "Equal!"
fi
Output:
Not Equal!