How to Multiply Variables in Bash
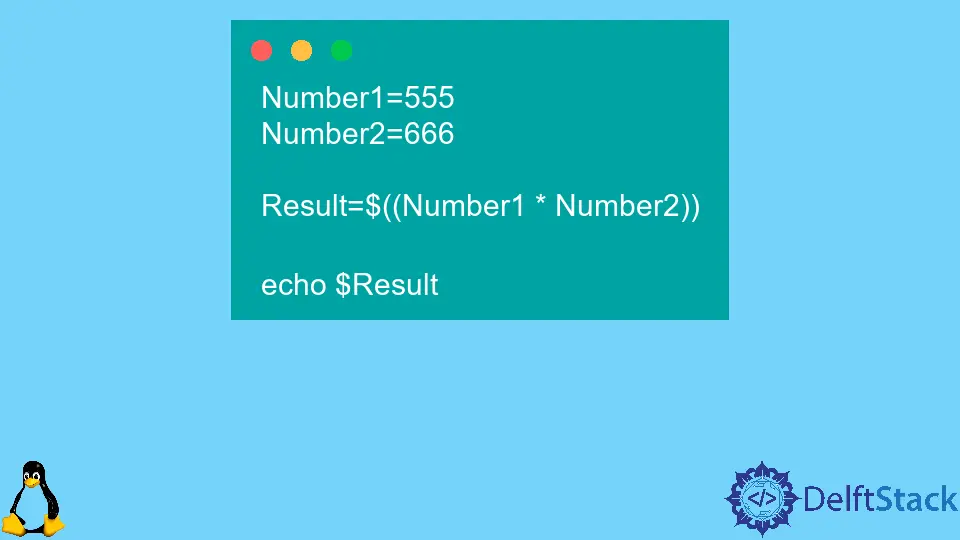
This tutorial demonstrates how to multiply two variables in Bash.
Multiply Variables in Bash
Multiplying two variables is an easy operation in Bash. We can use the arithmetic operator *
to multiply two numbers in Bash.
Multiplying is a step-by-step process in Bash. Follow the steps below to multiply two variables in Bash.
-
First of all, initialize two variables.
-
Then we multiply to variables using the
*
operator with$(...)
or the external program expressionexpr
. -
Make sure to assign the above multiplication to a variable.
-
Echo
the final variable.
Now let’s implement the variable multiplication based on the above steps.
Number1=555
Number2=666
Result=$((Number1 * Number2))
echo $Result
The code above will multiply the two variables, Number1
and Number2
, using the *
multiplication operator with $(...)
and then echo
the result. See the output:
369630
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook