How to Use the Mod Operator in Bash
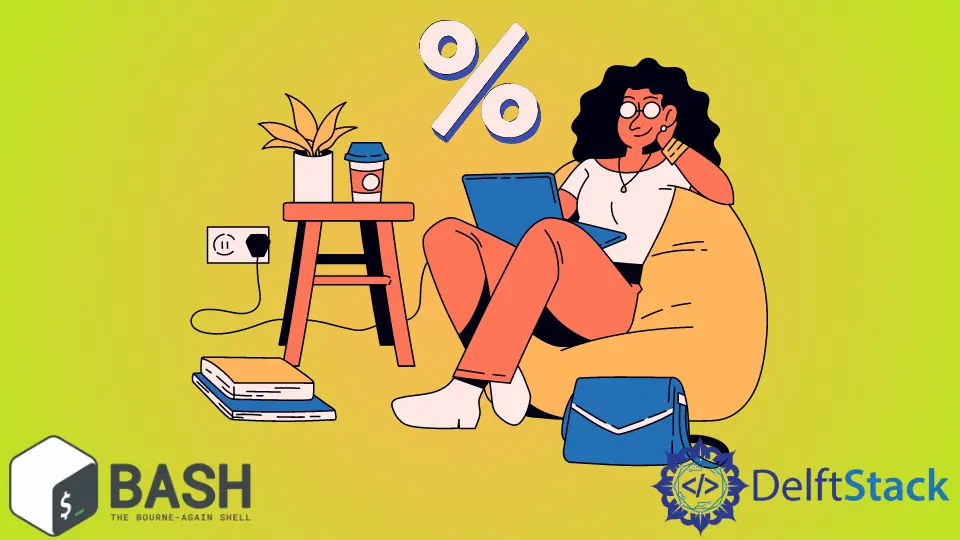
In this article, we will learn how to use the modulo (%
) operator in Bash.
Use the Mod (%
) Operator in Bash
The expr
command is what you need to use in Bash if you want to evaluate an expression. The expr
function must be called for each arithmetic operation to correctly assess the result derived from the expression.
To perform basic operations such as adding, subtracting, multiplying, dividing, and modulus on integers, we need to use the notation expr
.
Example:
It is necessary to run the expr
command to determine the value of the modulo variable before we can use it in the shell. Therefore, we have appended three expr
instructions in succession to determine the modulo of two integer values at each iteration by utilizing the percentage %
operator in between them.
We have obtained three remainder values as a result.
Example:
expr 25 % 2
expr 49 % 3
expr 67 % 7
Output:
1
1
4
Let us accept user input as parameters to make our code more interactive. This code uses two read
statements to obtain user input and save it in variables a
and b
; the input type must be an integer.
The remainder has been calculated using the res
variable and the modulo %
operator, resulting in the echo
command being executed.
Example:
read -p "Enter the first number: " a
read -p "Enter the second number: " b
res=$((a%b))
echo "The modulus of $a and $b is: $res"
Output:
Enter the first number: 8
Enter the second number: 3
The modulus of 8 and 3 is: 2
Another example:
for i in {1..10}
do
if [ $(expr $i % 2) != "0" ]; then
echo "$i"
fi
done
The code above lists all the odd numbers starting from 1 to 10.
Output:
1
3
5
7
9