How to Create an Infinite Loop in Bash
-
Method 1: Using the
while
Loop -
Method 2: Using the
until
Loop -
Method 3: Using the
for
Loop - Conclusion
- FAQ
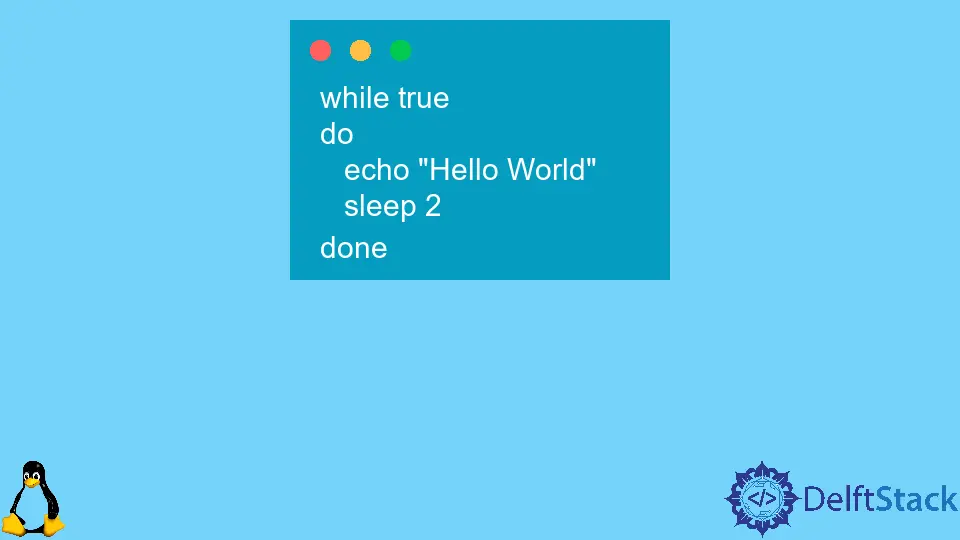
Creating an infinite loop in Bash can be a powerful tool for automation and scripting. Whether you’re looking to run a continuous process, monitor system resources, or simply keep a script running until manually stopped, understanding how to implement an infinite loop is crucial.
In this tutorial, we will explore various methods to create an infinite loop in Bash, with clear examples and explanations. By the end of this article, you will have a solid grasp of how to effectively use infinite loops in your Bash scripts, making your scripting experience more efficient and productive.
Method 1: Using the while
Loop
One of the most straightforward ways to create an infinite loop in Bash is by using the while
loop. This method checks a condition and continues executing as long as that condition is true. To create an infinite loop, you can simply use true
as the condition.
Here’s how you can do it:
while true; do
echo "This is an infinite loop"
sleep 1
done
In this example, the while true
statement creates a loop that will run indefinitely. Inside the loop, we use the echo
command to print a message to the terminal. The sleep 1
command pauses the loop for one second between iterations, making it easier to observe the output.
Output:
This is an infinite loop
This is an infinite loop
This is an infinite loop
...
This method is particularly useful for scripts that need to perform regular checks or tasks without stopping. You can easily terminate the loop by pressing Ctrl+C
, which sends an interrupt signal to the script.
Method 2: Using the until
Loop
Another way to create an infinite loop in Bash is by using the until
loop. This loop continues executing as long as the specified condition is false. To create an infinite loop, you can use a condition that is always false.
Here’s an example:
until false; do
echo "This is another infinite loop"
sleep 1
done
In this case, the until false
statement ensures that the loop runs indefinitely. Similar to the previous method, the echo
command outputs a message, and sleep 1
introduces a one-second delay.
Output:
This is another infinite loop
This is another infinite loop
This is another infinite loop
...
This method can be particularly useful when you want to perform operations until a specific condition becomes true. Like the while
loop, you can terminate it with Ctrl+C
.
Method 3: Using the for
Loop
You can also create an infinite loop using a for
loop by leveraging a sequence that never ends. Although this is less common, it’s still a valid approach.
Here’s how you can implement it:
for (( ; ; )); do
echo "This is an infinite for loop"
sleep 1
done
In this example, the for (( ; ; ))
syntax creates an infinite loop. The lack of initialization, condition, and increment means that the loop will run indefinitely. As before, the echo
command prints a message, and sleep 1
adds a delay.
Output:
This is an infinite for loop
This is an infinite for loop
This is an infinite for loop
...
This method is useful for scenarios where you might want to combine the capabilities of a for
loop with other operations. It’s important to remember that, like the previous methods, you can stop this loop with Ctrl+C
.
Conclusion
Creating an infinite loop in Bash is a simple yet powerful technique that can enhance your scripting capabilities. Whether you choose to use a while
, until
, or for
loop, understanding how to implement these loops allows you to automate tasks and monitor system activities effectively. Remember to use these loops responsibly, as they can consume system resources if not managed properly. With the knowledge gained from this tutorial, you can confidently incorporate infinite loops into your Bash scripts, making your automation tasks seamless and efficient.
FAQ
-
What is an infinite loop in Bash?
An infinite loop in Bash is a loop that continues to execute indefinitely until it is manually stopped or interrupted. -
How can I stop an infinite loop in Bash?
You can stop an infinite loop in Bash by pressingCtrl+C
, which sends an interrupt signal to the running script. -
Are infinite loops useful in scripting?
Yes, infinite loops can be very useful for tasks that require continuous monitoring or repeated actions, such as checking system status or running background processes. -
Can infinite loops cause issues in Bash scripts?
If not managed properly, infinite loops can consume system resources and lead to unresponsive scripts. It’s essential to ensure there’s a way to exit the loop or to implement conditions that will eventually break it. -
Can you nest infinite loops in Bash?
Yes, you can nest infinite loops in Bash, but be cautious as this can lead to complex and potentially unmanageable scripts if not carefully designed.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn