How to Include Script File in Another Bash Script
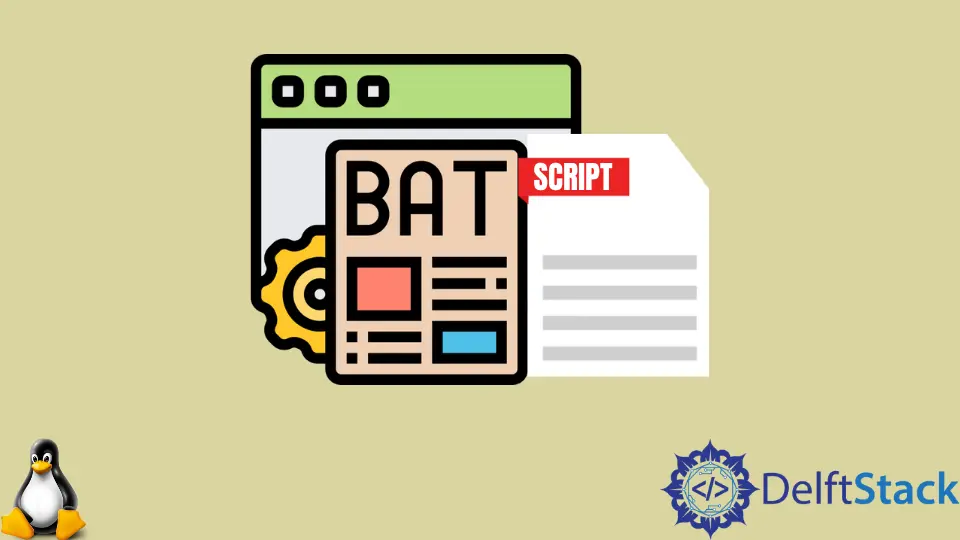
This article discusses different methods to include a Bash script file into another script file.
Include File in a Bash Script
Including or reusing a script is very simple in Bash. The source
keyword is similar to the #include
in C/C++.
To reuse a script, use the source
keyword with the name/full path file depending on whether you want to import the script from the same directory or another directory.
Generally, the syntax is given as follows:
source <filename>
Let’s say we have a script called sorting.sh
with the following content.
TEST_VAR="Hello"
The syntax would be as follows.
Bash Script:
#!/bin/bash
source sorting.sh
echo ${TEST_VAR}
Alternatively, the .
operator is a shorthand for the source
keyword and works similarly.
Bash Script:
#!/bin/bash
sorting.sh
echo ${TEST_VAR}
Both of the above examples will return the following output.
Output:
Hello
Note that the .
operator is POSIX compliant while the source
keyword is not. This means that the .
operator will work in all POSIX shell environments while the source
may not, but in the context of Bash, it is perfectly safe to use the source
keyword.
Another point to note is that source
does not work exactly like include
in C/C++ because it will execute the complete source script in the destination script rather than individually being able to call and execute separate functions.
The safest way to use a script in another script that will work with all levels of directories and will not cause errors because of directories being different or at different levels is using the following script.
Bash Script:
$(dirname "$0")/sorting.sh