How to Get Timestamp in Bash
- Using the date Command for Timestamps
- Using Git Commands for Timestamps
- Formatting Timestamps for Different Needs
- Using Timestamps in Git Hooks
- Conclusion
- FAQ
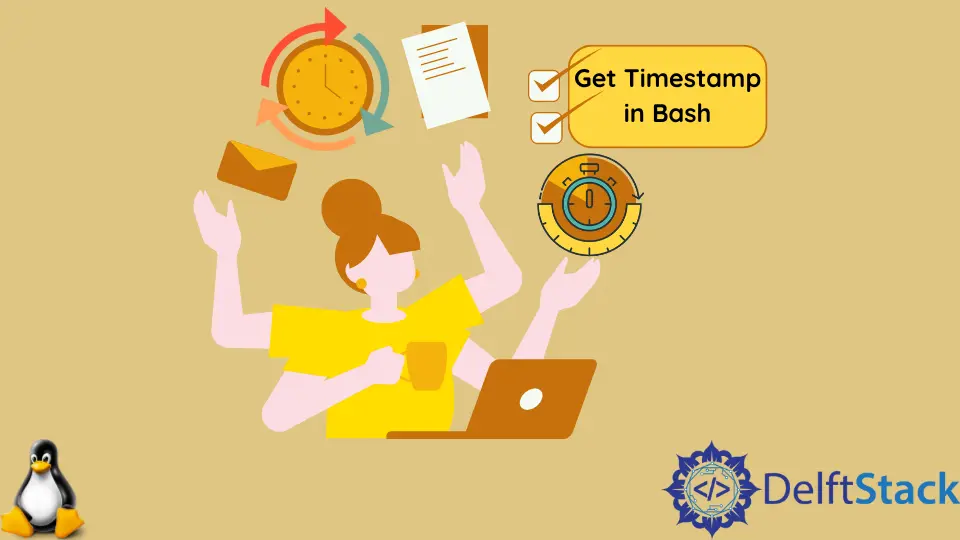
When working in a Bash environment, timestamps can be crucial for logging, file management, and version control. Whether you’re developing scripts or managing your Git repositories, knowing how to create and utilize timestamps can streamline your workflow.
In this article, we will delve into various methods to generate timestamps in Bash scripts. We’ll cover the essential Git commands that allow you to leverage timestamps effectively, along with examples that illustrate their practical applications. By the end of this guide, you’ll have a solid understanding of how to handle timestamps in your Bash scripts, enhancing your scripting capabilities and improving your overall productivity.
Using the date Command for Timestamps
One of the simplest ways to generate a timestamp in Bash is by using the date
command. This command is versatile and allows you to format the output as needed. Below is an example that shows how to create a timestamp variable in a Bash script.
timestamp=$(date +"%Y-%m-%d %H:%M:%S")
echo "Current Timestamp: $timestamp"
Output:
Current Timestamp: 2023-10-05 14:30:00
In this snippet, we use the date
command with the format specifier +"%Y-%m-%d %H:%M:%S"
. This format outputs the date and time in a human-readable format. The result is stored in the variable timestamp
, which can then be echoed to the console or used in further processing. This method is particularly useful for logging events or recording the time of file modifications in your scripts.
Using Git Commands for Timestamps
If you’re working within a Git context, you can also extract timestamps from commits. This is especially useful for tracking changes and understanding the history of your project. Here’s how to retrieve the timestamp of the last commit in a Git repository.
last_commit_timestamp=$(git log -1 --format=%cd)
echo "Last Commit Timestamp: $last_commit_timestamp"
Output:
Last Commit Timestamp: Thu Oct 05 14:30:00 2023 -0400
In this example, the git log
command is used to fetch the timestamp of the most recent commit. The -1
option limits the output to the last commit, and --format=%cd
specifies that we want the commit date. The result is stored in the variable last_commit_timestamp
, which can be used for logging or notifications. This method is particularly beneficial when you want to automate processes based on commit history.
Formatting Timestamps for Different Needs
Sometimes, you may need timestamps in different formats for various applications. The date
command allows you to customize the output format easily. Here’s an example that shows how to create a timestamp in a different format.
custom_timestamp=$(date +"%d-%m-%Y %I:%M %p")
echo "Custom Formatted Timestamp: $custom_timestamp"
Output:
Custom Formatted Timestamp: 05-10-2023 02:30 PM
In this code, we use the date
command again, but this time with a different format specifier: +"%d-%m-%Y %I:%M %p"
. This format outputs the date in a day-month-year format along with the time in a 12-hour format, which may be more suitable for certain applications. By adjusting the format specifiers, you can easily tailor the timestamp to meet your specific requirements.
Using Timestamps in Git Hooks
Git hooks are scripts that run automatically at certain points in the Git workflow. You can integrate timestamps into these hooks to log activities and changes. Here’s an example of a pre-commit hook that logs the commit timestamp to a file.
#!/bin/bash
echo "Commit Timestamp: $(date +"%Y-%m-%d %H:%M:%S")" >> commit_log.txt
Output:
Commit Timestamp: 2023-10-05 14:30:00
In this example, we create a simple pre-commit hook that appends the current timestamp to a file named commit_log.txt
each time a commit is made. This can help maintain a record of when changes were made and can be invaluable for debugging or auditing purposes. To use this, you would place the script in the .git/hooks/
directory and give it executable permissions.
Conclusion
Timestamps are a vital component of any Bash scripting and Git workflow. By using simple commands like date
and various Git commands, you can generate, format, and utilize timestamps effectively. Whether you’re logging events, tracking changes in your Git repository, or automating processes, mastering timestamps will enhance your scripting skills and improve your overall productivity. With the methods outlined in this article, you now have the tools to implement timestamps in your Bash scripts seamlessly.
FAQ
-
How do I create a timestamp in a Bash script?
You can create a timestamp in a Bash script using thedate
command. For example,timestamp=$(date +"%Y-%m-%d %H:%M:%S")
generates a formatted timestamp. -
Can I get the timestamp of the last Git commit?
Yes, you can usegit log -1 --format=%cd
to retrieve the timestamp of the last commit in your Git repository. -
How can I format the timestamp differently?
You can customize the output of thedate
command by using different format specifiers. For example,date +"%d-%m-%Y %I:%M %p"
gives you a different format.
-
What are Git hooks, and how can I use timestamps in them?
Git hooks are scripts that run at specific points in the Git workflow. You can use timestamps in hooks to log activities, like appending the current timestamp to a log file during a commit. -
Are there any best practices for using timestamps in Bash scripts?
Always use consistent formatting for timestamps and consider time zones if your scripts will run in different locales. This ensures clarity and avoids confusion.