How to Solve Export Not a Valid Identifier Error in Bash
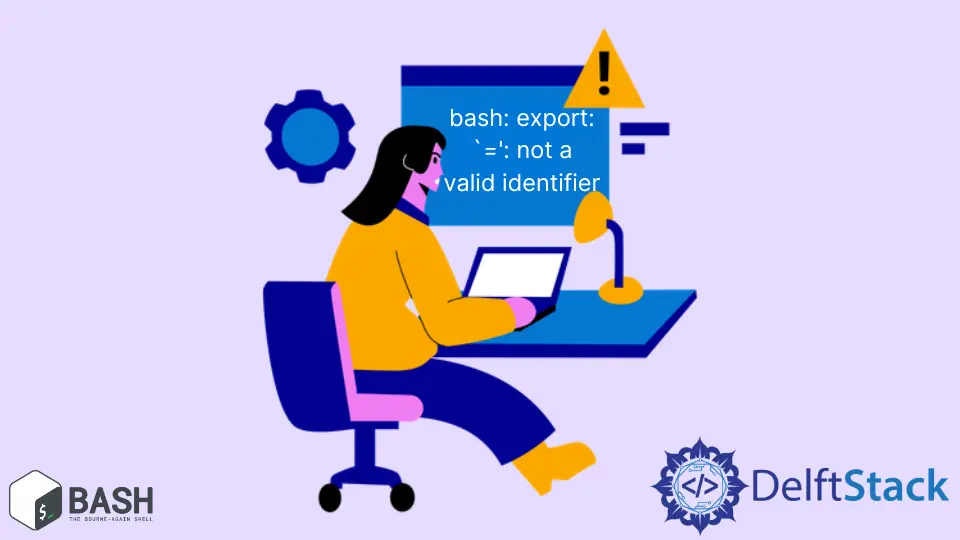
We can use the export
command to add the variable to the environment variables list of the shell. This article will explain how to export variables correctly and solve the bash: export: `=': not a valid identifier
error in Bash.
Solve bash: export: `=': Not a Valid Identifier
Error in Bash
The export
command exports attribute to variables corresponding to the specified names in the shell. The variable will be available in the environment of subsequently executed commands.
If the variable name is followed by =word
, the variable value is set to word
.
We can use the export
command like below.
export variable=attribute
Make sure you do not leave any spaces between words. You will get the bash: export: `=': not a valid identifier
error using the command below.
export variable = attribute
Also, review the .bashrc
and .bash_profile
files. If this command is used with spaces in these files, the error will occur.
For example, the PATH
can be specified with the following command in these files.
export PATH = $PATH:/usr/local/bin
We can fix the error by deleting the space characters here.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn