Multiline Echo in Bash
- Understanding Multiline Echo in Bash
-
Method 1: Using the
echo
Command with Newline Characters - Method 2: Using Here Documents
-
Method 3: Using the
printf
Command - Method 4: Using an Array
- Conclusion
- FAQ
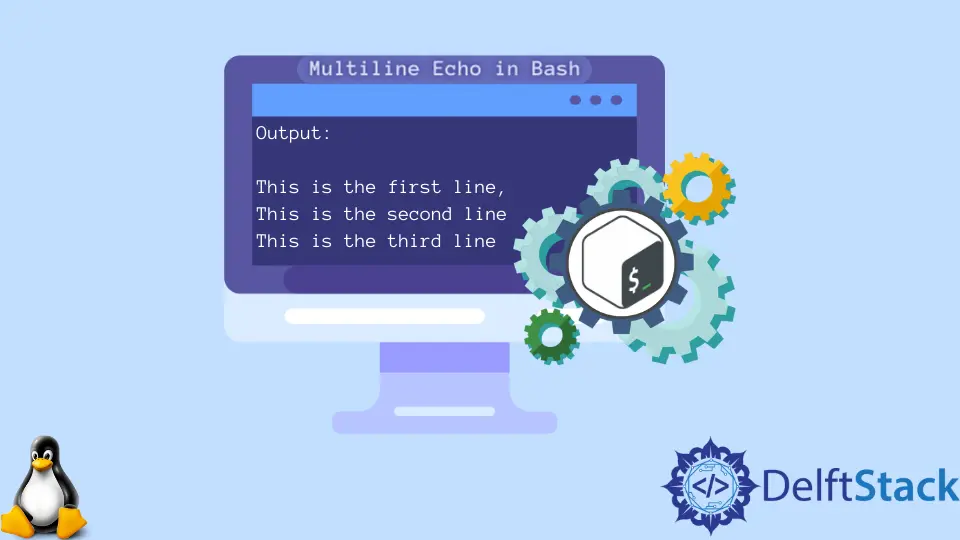
When working in Bash, you may often find yourself needing to output multiple lines of text. Whether you’re creating scripts or just experimenting in the terminal, the ability to echo multiple lines can be incredibly useful.
This tutorial will discuss how to effectively implement multiline echo in Bash. We’ll explore different methods, share practical examples, and guide you through the nuances of each approach. By the end of this article, you’ll be equipped with the knowledge to enhance your Bash scripting skills and streamline your workflow. Let’s dive in!
Understanding Multiline Echo in Bash
In Bash, the echo
command is primarily used to display text or variables. However, when it comes to multiline output, there are several techniques you can use. The simplest way to achieve multiline output is through the use of newline characters, but there are also other methods that can make your scripts cleaner and more readable. Below, we’ll explore these methods in detail.
Method 1: Using the echo
Command with Newline Characters
One of the most straightforward methods for creating multiline output in Bash is by using the echo
command along with newline characters. You can insert \n
to indicate where a new line should begin. However, you need to enable the interpretation of backslash escapes by using the -e
option with echo
.
Here’s how you can do this:
echo -e "This is the first line.\nThis is the second line.\nThis is the third line."
Output:
This is the first line.
This is the second line.
This is the third line.
In this example, the -e
option allows the echo
command to interpret the \n
characters as new lines. This results in a clean output spread across multiple lines. This method is particularly useful for quick scripts where you need to output a few lines of text without much formatting. However, if your output becomes more complex, you might want to explore other methods.
Method 2: Using Here Documents
Another effective way to achieve multiline echo in Bash is by using Here Documents. This method allows you to create a block of text that can be processed as a single unit. It’s especially useful for longer strings of text or when you want to include variables or commands within the output.
Here’s how to use Here Documents:
cat << EOF
This is the first line.
This is the second line.
This is the third line.
EOF
Output:
This is the first line.
This is the second line.
This is the third line.
In this example, cat
is used with a Here Document, which begins with << EOF
and ends with EOF
. Everything in between is treated as input to the cat
command and is printed as output. This method is not only clean and readable but also allows for easy editing of the text block. You can even include variables and commands inside the Here Document, making it a powerful tool for more complex scripts.
Method 3: Using the printf
Command
The printf
command is another great alternative for producing multiline output in Bash. Unlike echo
, printf
provides more control over formatting and is better suited for complex output scenarios. You can specify format strings and control the output more precisely.
Here’s how to use printf
for multiline output:
printf "This is the first line.\nThis is the second line.\nThis is the third line.\n"
Output:
This is the first line.
This is the second line.
This is the third line.
In this example, printf
is used with newline characters similar to the first method. However, printf
can also format numbers, strings, and other types of data, making it a versatile choice for various output needs. The control it offers over formatting is particularly beneficial when you need to align text or display data in a specific way.
Method 4: Using an Array
If you have multiple lines of text stored in an array, you can easily echo each element of the array in a loop. This approach is useful when you want to manage your output dynamically.
Here’s how to use an array for multiline output:
lines=("This is the first line." "This is the second line." "This is the third line.")
for line in "${lines[@]}"; do
echo "$line"
done
Output:
This is the first line.
This is the second line.
This is the third line.
In this code snippet, we define an array called lines
containing three strings. The for
loop iterates over each element in the array and echoes it to the terminal. This method is particularly useful when dealing with a variable number of lines or when the lines are generated dynamically during script execution.
Conclusion
In this tutorial, we’ve explored various methods for achieving multiline echo in Bash. From using the echo
command with newline characters to employing Here Documents, printf
, and arrays, each method has its own advantages and use cases. Depending on your specific needs, you can choose the approach that best suits your scripting style. Mastering these techniques will not only enhance your Bash scripting skills but also improve the readability and maintainability of your scripts. Happy scripting!
FAQ
-
What is the difference between
echo
andprintf
in Bash?
echo
is simpler and primarily used for basic output, whileprintf
offers more control over formatting and is better for complex outputs. -
Can I use variables in Here Documents?
Yes, you can include variables in Here Documents, and they will be expanded when the document is processed. -
Is there a limit to how many lines I can echo in Bash?
There is no strict limit, but practical limits may arise from system memory or terminal display size. -
How do I include special characters in my output?
You can escape special characters using a backslash (\
) or use single quotes to prevent interpretation. -
Can I redirect the output of multiline echo to a file?
Yes, you can redirect the output of any of these methods to a file using the>
operator.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn