How to Find the Current Folder Name in Bash
- Using the pwd Command
- Using the Command Substitution
- Using the git rev-parse Command
- Using the git status Command
- Conclusion
- FAQ
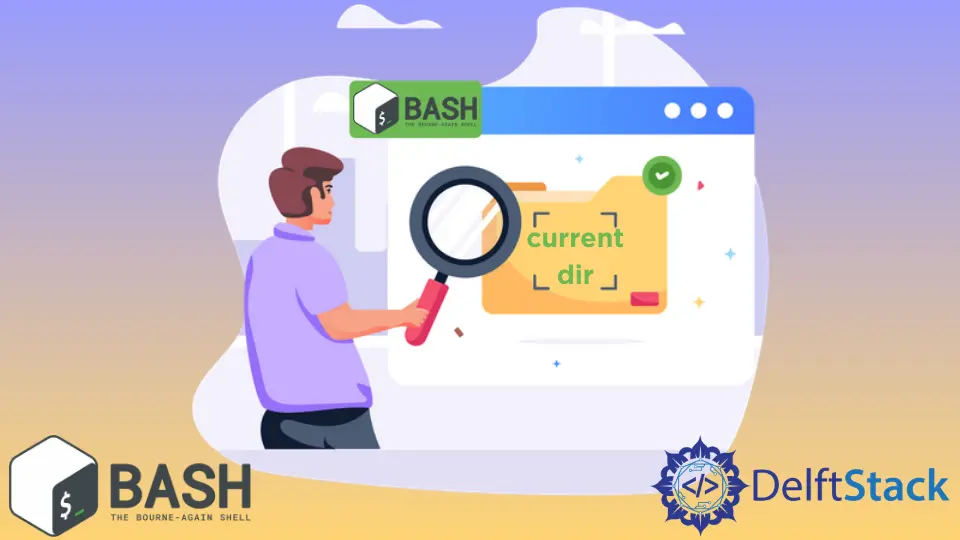
When working in a Bash environment, knowing how to find the current folder name can be incredibly useful. Whether you’re navigating through directories, managing files, or using version control with Git, having quick access to your working directory’s name helps streamline your workflow.
In this tutorial, we will explore different methods to find the current folder name using Bash commands. You’ll learn practical techniques that can enhance your command-line skills and improve your productivity. So, let’s dive into the various ways to discover the current folder name in Bash.
Using the pwd Command
One of the simplest and most straightforward ways to find the current folder name in Bash is by using the pwd
command. This command stands for “print working directory,” and it displays the full path of the current directory you’re in. To extract just the folder name from this path, you can combine pwd
with other commands.
Here’s how you can do it:
current_folder=$(basename "$(pwd)")
echo $current_folder
Output:
example_folder
In this example, pwd
retrieves the full path of the current directory. The basename
command then takes that path and strips away everything except for the final folder name. By assigning the result to the variable current_folder
, you can easily echo it out or use it in your scripts. This method is efficient and works seamlessly in any Bash environment.
Using the Command Substitution
Another effective way to find the current folder name is by utilizing command substitution in Bash. This technique allows you to capture the output of a command and use it as an input for another command. Here’s how you can achieve this:
echo $(basename "$PWD")
Output:
example_folder
In this command, "$PWD"
is an environment variable that always contains the path of the current working directory. By wrapping it with basename
, you can directly print the current folder name to the terminal. This method is particularly useful for quick checks without needing to assign values to variables. It’s a quick and efficient way to get the job done.
Using the git rev-parse Command
If you’re working within a Git repository, you might want to find the current folder name in the context of your Git project. The git rev-parse
command can be particularly useful here. It provides various information about the repository, including the current branch and, in this case, the current folder name.
Here’s how you can do it:
current_folder=$(basename "$(git rev-parse --show-toplevel)")
echo $current_folder
Output:
example_repo
In this example, git rev-parse --show-toplevel
returns the absolute path to the root of the Git repository. By applying basename
, you can extract just the repository’s folder name. This is particularly handy when you’re working in nested directories within a Git project and need to keep track of your current folder’s context.
Using the git status Command
Another Git-specific method to find the current folder name is by using the git status
command. While this command is typically used to check the status of your repository, it can also provide the current directory context. Here’s how you can implement it:
current_folder=$(git status | head -n 1 | awk '{print $3}')
echo $current_folder
Output:
example_folder
In this command, git status
provides a summary of the current state of your Git repository. The head -n 1
command extracts the first line of this output, which typically contains the current folder name. The awk
command is then used to print the third word from that line, which should be the folder name. This method is slightly more complex but can be very useful in certain situations where you want to gather additional information from your Git status.
Conclusion
Finding the current folder name in Bash is a fundamental skill that can greatly enhance your command-line efficiency. Whether you choose to use simple commands like pwd
and basename
or delve into Git-specific commands like git rev-parse
, each method has its own advantages. By incorporating these techniques into your workflow, you can navigate your file system and manage your projects more effectively. Now that you have a variety of methods at your disposal, you can choose the one that best fits your needs.
FAQ
- What is the pwd command used for?
Thepwd
command is used to print the current working directory in a Bash environment.
-
How can I find the current folder name in a Git repository?
You can use commands likegit rev-parse --show-toplevel
orgit status
to find the current folder name within a Git repository. -
Can I use these commands in scripts?
Yes, all the commands discussed can be used in Bash scripts to automate tasks involving folder navigation. -
What does the basename command do?
Thebasename
command extracts the final portion of a file path, which is typically the folder or file name. -
Is there a way to find the folder name without using Git?
Absolutely! You can simply use thepwd
andbasename
commands to find the current folder name without any Git context.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn