How to Check Exit Code in Bash
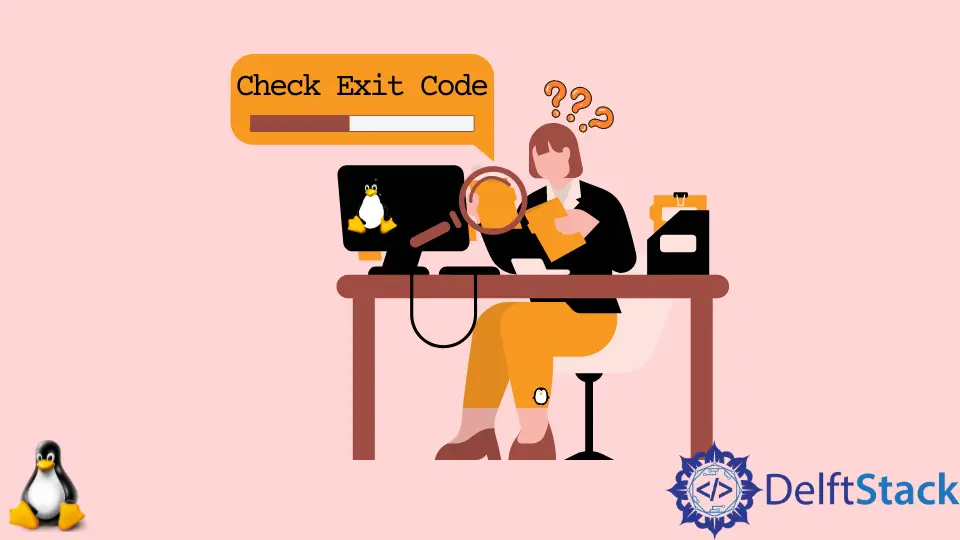
This tutorial will describe several methods for getting and displaying the exit status of a statement in Bash. We will first discuss using a simple if
statement to get the exit status, after which we will discuss alternatives and shorthand notations for getting the exit status.
Check Exit Code in Bash
The exit status is essentially an integer that tells us whether or not the command succeeded. Non-intuitively, an exit status of 0
indicates success, and any non-zero value indicates failure/error.
This non-intuitive solution allows for different error codes to represent different errors. For example, if a command is not found, then the exit status of 127
will be returned.
The way to check the exit code in Bash is by using the $?
command. If you wish to output the exit status, it can be done by:
# your command here
testVal=$?
echo testVal
We know that non-zero exit codes are used to report errors. If we want to check for errors in general, we can write a small script with the command in question and an if
statement that checks whether the exit code is of an error (non-zero) or if the exit code indicates proper execution.
# your command here
testVal=$?
if [$testVal -ne 0]; then
echo "There was some error"
fi
exit $testVal
A shorthand notation can be done using a test
on the $?
command. A minimal example of this code can be:
# your command here
test $? -eq 0 || echo "There was some error"
For a better understanding of the above statement, we can expand it.
# your command here
exitStat = $?
test $exitStat -eq 0 && echo "No error! Worked fine" || echo "There was some error";
exit $exitStat
Above, we can see the "No error! Worked fine"
message in case of successful execution, and the error message in case of failure.
Important Notes and Possible Alternate Solutions
If exit codes are used to see whether or not the command is executed correctly, we have a much simpler alternative.
# define your_command here
# your_command = enter a command here
if your_command; then
echo command returned true
else
echo command returned an error
fi
In this case, we generally get the same output as we would using the first couple of options. Furthermore, getting specific information on the exit code or getting the specific exit code requires running $?
manually.
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn