How to Solve cd: Too Many Arguments Error in Bash
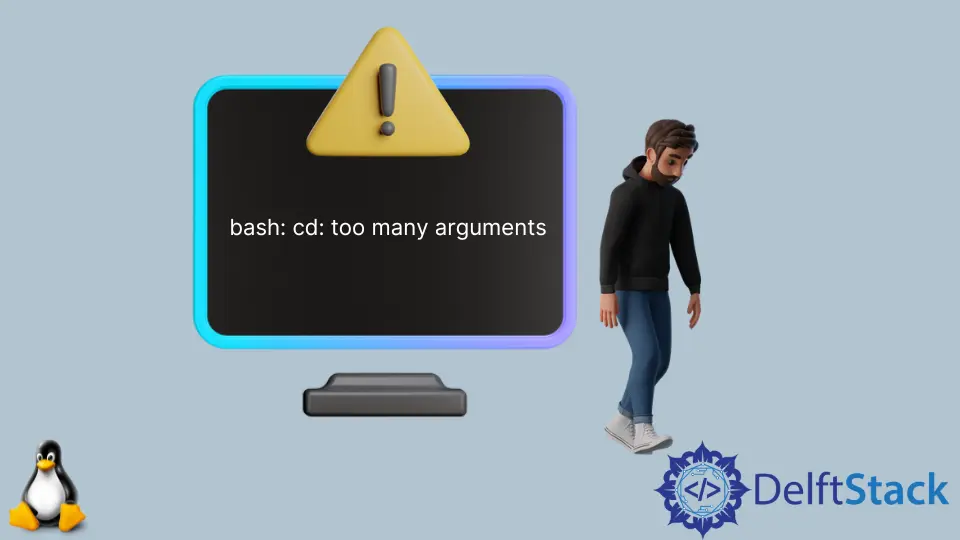
We use the cd
command to change the working directory in Linux. However, incorrect use of this command may cause an error.
This article will explain how to solve the bash: cd: too many arguments
error in Linux Bash.
Solve bash: cd: too many arguments
Error in Bash
The cd
command accepts a directory name as an argument and replaces the current shell environment’s working directory with the given directory.
If no argument is given, the cd
command behaves as if the directory named in the HOME
environment variable was specified as the argument.
If the directory you want to go to consists of more than one word and contains spaces, you should not give it an argument directly. The command below will generate the bash: cd: too many arguments
error.
cd test directory
The command accepts test
and directory
as separate arguments and cannot operate. To solve this problem, you must write the directory name in quotation marks; use single or double quotes as you wish.
The following command will run without error, and the current directory will be replaced with the test directory
. Using a slash (/
) after the directory name is optional.
cd "test directory/"
Another way to do this is to use the backslash character. The backslash (\
) is an escape character, and it preserves the literal value of the next character that follows.
So you can use the space character.
The following command will also run without error, and the current directory will be replaced with the test directory
. Using a slash (/
) after the directory name is optional.
cd test\ directory/
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn