How to Call Another Script From Current Script in Bash
- Create a Bash Script
-
Use the
source
Command to Call Another Script From the Current Script in Bash -
Use the
.
Symbol to Call Another Script From the Current Script in Bash -
Use the
sh
Command to Call Another Script From the Current Script in Bash
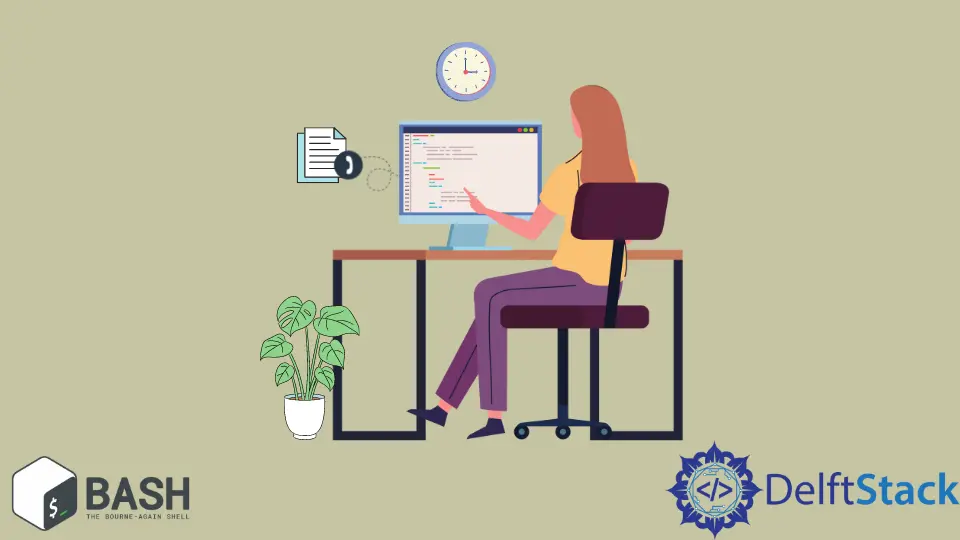
Sometimes we need to run an external script from the current running script. Bash enables us to run an external script from another script by calling.
There are three easy methods of calling an external script: the source
command, the symbol .
, and the sh
command. You can choose any one of these methods.
In this article, we will see how we can call an external Bash script from the current running script. Also, we will look at some examples and explanations to make the topic easier.
Create a Bash Script
Before starting, suppose we designed our first Bash script named ScriptOne.sh
with the below code:
echo 'This is a first bash script that is waiting for a response'
We need to design another Bash script that will refer to our first Bash script. We can call the first script from another Bash script in three ways described below.
Use the source
Command to Call Another Script From the Current Script in Bash
In our first method, we will use the command source
. It is a special command that can be used to execute another script.
Using this command, the code for our example will be like the following:
echo 'This is the second file that will call another script'
source first. sh
Please note that there should be a space after the source
command.
Use the .
Symbol to Call Another Script From the Current Script in Bash
This is the simplest method. In this method, we will be using the symbol .
.
It will exactly work like our above method. The code for the same example using the symbol .
will be as follows:
echo 'This is the second file that will call another script'
. first. sh
Please note that there should be a space after the .
symbol.
Use the sh
Command to Call Another Script From the Current Script in Bash
In our last example, we will use the command sh
, a built-in Bash command that can be used to execute external scripts. The code for the same example using the sh
command will be as follows:
echo 'This is the second file that will call another script'
sh first. sh
Please note that there should be a space after the sh
command.
All of the methods shared above will work in the same way. After executing any one of the method examples, you will get an output as follows:
This is the second file that will call another script
This is a first bash script that is waiting for a response
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn