How to Solve Bad Substitution Error in Bash
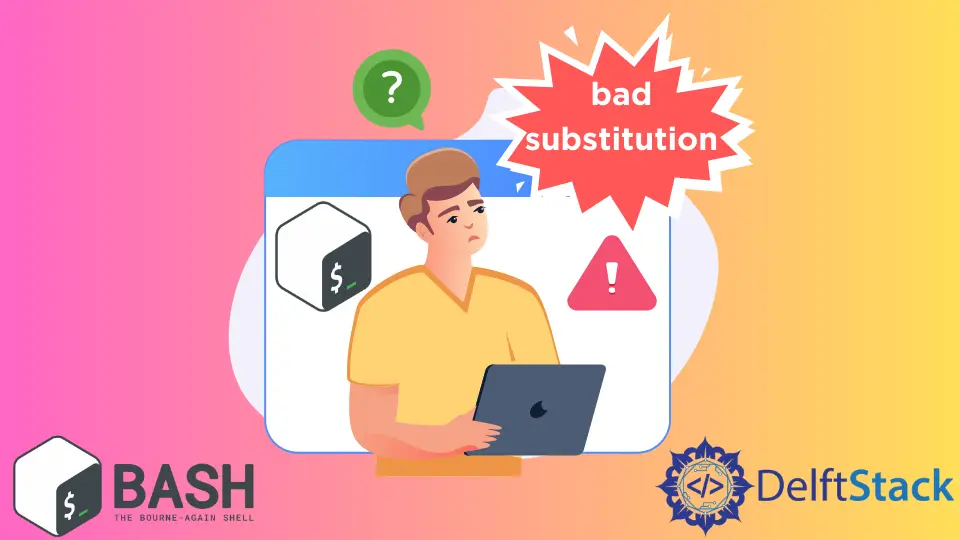
Variable substitution can be used to manipulate the value of a variable in Bash scripting. However, this feature is not available in every shell.
This article will explain how to solve the bad substitution
error in Linux Bash.
Solve Bad Substitution
Error in Bash
We can perform various operations by obtaining substrings from variables with variable substitution. Let’s examine the sample Bash script below.
The first line is called shebang. It is used to tell the operating system which shell to use to parse the file.
We define a string variable named str
in the second line. Then we assign the hello
and world
substrings to separate variables.
It looks like a completely correct code.
#!/bin/sh
str="hello world"
hello=${str:0:5}
world=${str:6}
But when we execute this code, we get a Bad substitution
error. Because in Ubuntu, /bin/sh
points to the dash shell.
Unfortunately, the dash shell does not have a variable substitution feature.
You can see which shell the /bin/sh
points to with the following commands.
which sh
ls -l /bin/sh
As you see, /bin/sh
is a symbolic link that points to /bin/dash
. To change the shell used and solve the bad substitution
error, we must first change the first line of code to #!/bin/bash
.
So, the code is no longer executed with dash but with bash.
However, you should still avoid executing the file with the sh file.sh
command after adding this statement. The #!/bin/bash
line will be ignored if you do this.
It is only used when you execute your script directly.
You can execute the file with the ./file.sh
or bash file.sh
command. You will no longer get this error.
Yahya Irmak has experience in full stack technologies such as Java, Spring Boot, JavaScript, CSS, HTML.
LinkedIn