How to Get Array Length in Bash
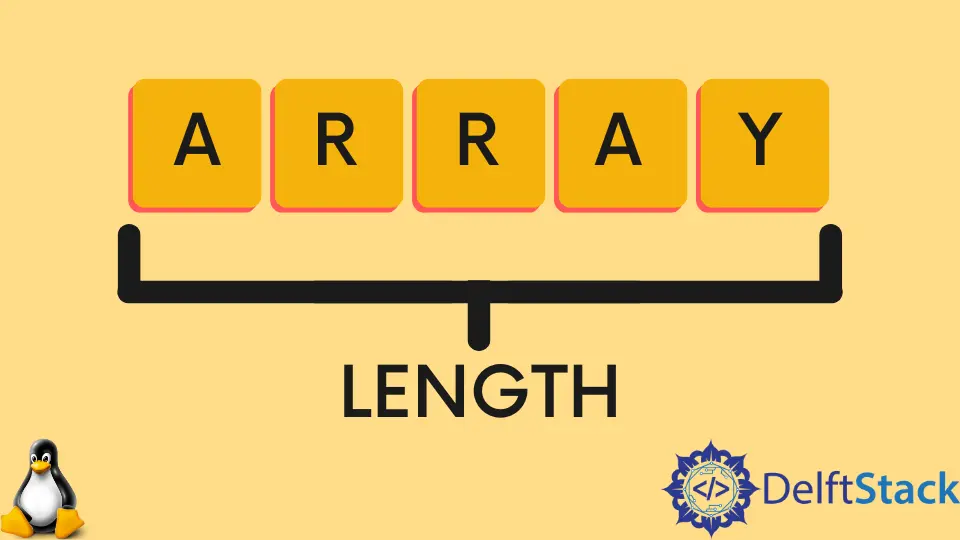
We need to know the length of the array for various purposes. For example, if you are looking for specific data in an array and don’t know the array’s length, you need to find the array’s length first before starting the search.
There is a built-in function or keyword for other programming languages to find the array length. But in Bash scripting, it’s a bit different.
In this article, we will see how we can find the array length and use it for various purposes.
Get the Array Length in Bash
The general syntax for finding the length of an array is:
${#ARRAY[*]}
In the below example, we are only finding the length of the array:
names=("Alen" "Walker" "Miller")
echo The length of the array is ${#names[*]}
In the above code, we counted the length of the names
array. You will get the below output after you run the example code.
Output:
The length of the array is 3
Get the Array Length With the for
Loop in Bash
Now, it’s time to go on an advance example. We have successfully understood how to find the length of an array.
We will now see how we can use this array length for various purposes. Below, we shared the updated version of our previous example, which will first count the length of the array and then use it in a for
loop to show all the elements inside the array.
This is the code for our example:
names=("Alen" "Walker" "Miller")
len=${#names[*]}
echo The length of the array is - $len
for (( i=0; i<$len; i++ ))
do
echo The value of element $i is: ${names[$i]}
done
As you can see, in the line len=${#names[*]}
, we created a variable named len
and assigned it to the value of the array’s length. It’s important because we need to know the array length to run a loop and extract array data.
After that, we printed the array length and ran a for
loop to extract each array element. If you look at the below output of the program, you can see we started the array index from 0
.
As we know, the array index always starts from 0
.
Output:
The length of the array is 3
The value of element 0 is: Alen
The value of element 1 is: Walker
The value of element 2 is: Miller
Please note that all the code used in this article is written in Bash. It will only work in the Linux Shell environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn