How to Assign Default Value in Bash
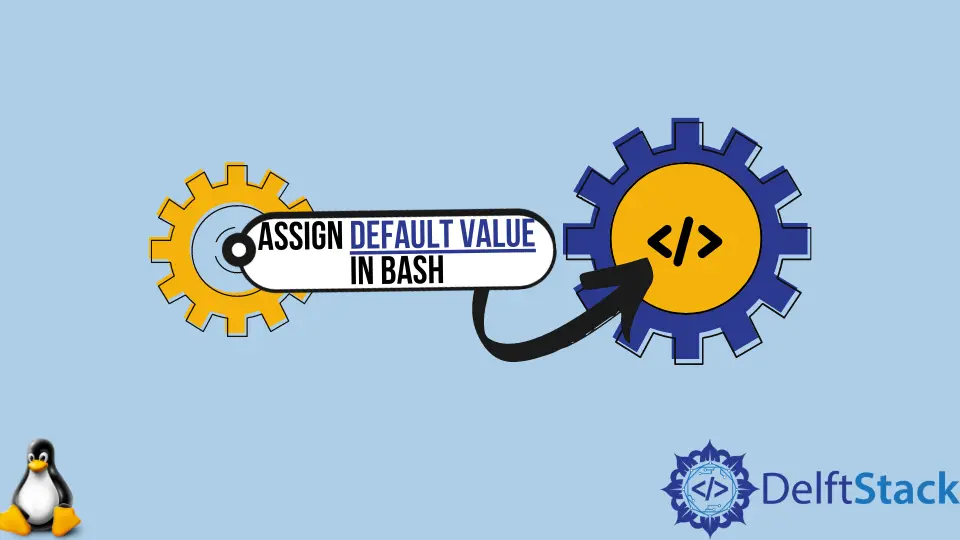
This article will introduce the approaches used for providing a default value to the variables in bash scripts.
Provide a Default Value to a Variable in a Bash Script
The basic approach we follow while providing a default value in a bash script is below.
variable={$variable:-value}
But we can use a better short-hand form of this by using colon at the beginning.
: ${variable:=value}
The colon at the beginning ignores the arguments.
Use ${variable-value}
or ${variable:-value}
echo ${greet-hello}
echo ${greet:-hello}
greet=
echo ${greet-hello}
echo ${greet:-hello}
Output:
hello
hello
hello
The key difference between using ${greet-hello}
and ${greet:-hello}
is that ${greet-hello}
will use the default value hello
if the variable greet
was never set to a value. On the other hand, ${greet:-hello}
will use default value either if the variable was never set a value or was set to null i.e. greet=
.
Use ${variable:-value}
or ${variable:=value}
echo ${greet:-Hello}
echo ${greet:-Namaste}
echo ${greet:=Bonjour}
echo ${greet:=Halo}
Output:
Hello
Namaste
Bonjour
Bonjour
Using :-
will substitute the variable with the default value, whereas :=
will assign the default value to the variable.
In the given example,
${greet:-Namaste}
prints outNamaste
as${greet:-Hello}
has substituted thegreet
variable with default value as it was not set.${greet:=Bonjour}
will set the value ofgreet
toBonjour
as its value was never set.${greet:=Halo}
will not use the default valueHalo
as the variablegreet
was set value previously i.e.Bonjour
.