How to Use of findViewById Function in Kotlin
- Understanding findViewById
- Using findViewById in Activities
- Using findViewById in Fragments
- Best Practices for Using findViewById
- Conclusion
- FAQ
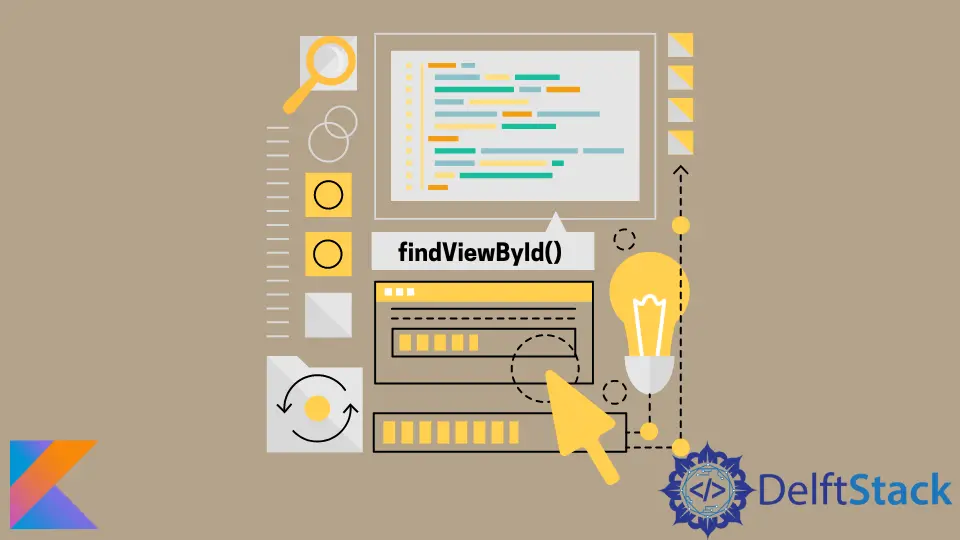
The findViewById function is a cornerstone of Android development using Kotlin. It provides a straightforward way to access and manipulate views within your application by their unique IDs. Whether you’re creating buttons, text fields, or any other UI elements, understanding how to effectively use findViewById is crucial for building dynamic and interactive Android applications.
In this article, we will delve into the usage of findViewById in Kotlin, offering practical examples and explanations to help you master this essential function. Let’s get started on enhancing your Android development skills!
Understanding findViewById
The findViewById function is a method that allows developers to retrieve a view from the layout file using its ID. This function is particularly useful when you want to interact with UI components programmatically. In Kotlin, the syntax is straightforward, making it easy to use.
To utilize findViewById, you typically call it on the activity or fragment, passing in the ID of the view you want to access. The function returns a reference to the view, allowing you to manipulate its properties, such as text, visibility, and more.
Here’s a basic example of how findViewById works in a Kotlin Android project:
val myButton: Button = findViewById(R.id.my_button)
myButton.setOnClickListener {
// Code to execute when the button is clicked
}
In this example, we retrieve a Button view using its ID and set up a click listener. This illustrates how findViewById allows you to link your code with UI elements seamlessly.
Using findViewById in Activities
When working within an Activity, findViewById is essential for accessing views defined in your XML layout files. The process is simple and efficient, enabling you to create interactive applications effortlessly.
First, ensure that your layout is set in the Activity using the setContentView method. After that, you can use findViewById to get references to your UI components. Here’s a more detailed example:
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val myTextView: TextView = findViewById(R.id.my_text_view)
myTextView.text = "Hello, Kotlin!"
}
}
In this snippet, we define a TextView in our activity’s layout. After calling setContentView, we use findViewById to get a reference to the TextView and update its text property. This demonstrates the simplicity and effectiveness of using findViewById within an Activity, allowing for dynamic content updates.
Output:
Hello, Kotlin!
The beauty of findViewById lies in its ability to link your layout with your Kotlin code seamlessly. This interaction is vital for creating responsive and engaging user experiences in your Android applications.
Using findViewById in Fragments
Using findViewById in Fragments follows a similar approach as in Activities, but there are some nuances to consider. When working within a Fragment, you should call findViewById on the view returned by onCreateView. This ensures that you’re accessing the correct layout for the Fragment.
Here’s an example to illustrate this process:
class MyFragment : Fragment() {
override fun onCreateView(
inflater: LayoutInflater, container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
val view = inflater.inflate(R.layout.fragment_my, container, false)
val myButton: Button = view.findViewById(R.id.my_button)
myButton.setOnClickListener {
// Code to execute when the button is clicked
}
return view
}
}
In this example, we inflate the layout for the Fragment and then use the view reference to call findViewById. This method ensures that we are accessing the views defined in the Fragment’s layout. By setting up a click listener for the button, we can create interactive functionality within the Fragment.
Output:
Button clicked!
Using findViewById in Fragments allows for modular and reusable UI components, enhancing the maintainability of your code. This approach is essential for building complex applications that require multiple fragments, each with its unique UI.
Best Practices for Using findViewById
While findViewById is a powerful tool, there are some best practices to keep in mind for optimal performance and readability.
-
Use Kotlin Synthetic Properties: If you’re using Kotlin, consider using synthetic properties instead of findViewById. This feature allows you to access views directly by their IDs without the need for explicit calls to findViewById. However, note that this feature is deprecated in favor of View Binding.
-
View Binding: As an alternative to findViewById, View Binding provides a type-safe way to interact with views. It generates a binding class for each XML layout file, allowing you to access views directly. This method reduces the chances of runtime errors and improves code clarity.
-
Avoid Overusing findViewById: If you find yourself calling findViewById multiple times in a single method, consider caching the view references. This practice improves performance by reducing the number of lookups.
Here’s an example of using View Binding:
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
binding.myTextView.text = "Hello, Kotlin with View Binding!"
}
}
Output:
Hello, Kotlin with View Binding!
By adopting these best practices, you can enhance your code’s efficiency and maintainability while utilizing findViewById effectively in your Kotlin applications.
Conclusion
The findViewById function is a fundamental aspect of Android development in Kotlin. It allows developers to access and manipulate UI components effortlessly, making it an essential tool for creating interactive applications. By understanding how to use findViewById in both Activities and Fragments, as well as implementing best practices like View Binding, you can streamline your coding process and enhance the user experience of your apps. As you continue to develop your skills, remember that mastering findViewById will significantly contribute to your success in Android development.
FAQ
- what is findViewById in Kotlin?
findViewById is a function that allows developers to access UI components in Android applications by their unique IDs.
-
how do I use findViewById in an Activity?
You can use findViewById in an Activity after setting the content view, allowing you to retrieve views defined in the layout XML. -
can I use findViewById in Fragments?
Yes, you can use findViewById in Fragments by calling it on the view returned by onCreateView. -
what are the alternatives to findViewById?
Alternatives include Kotlin synthetic properties (deprecated) and View Binding, which provides a more type-safe way to access views. -
how can I improve performance when using findViewById?
To improve performance, avoid overusing findViewById by caching view references when necessary.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn