Ternary Conditional Operator in Kotlin
- The Ternary Operator in Kotlin
-
Use
if-else
in Kotlin -
Use
if-else-if
Ladder in Kotlin -
Use
when
Expression in Kotlin -
Use Elvis Operator (
?:
) in Kotlin
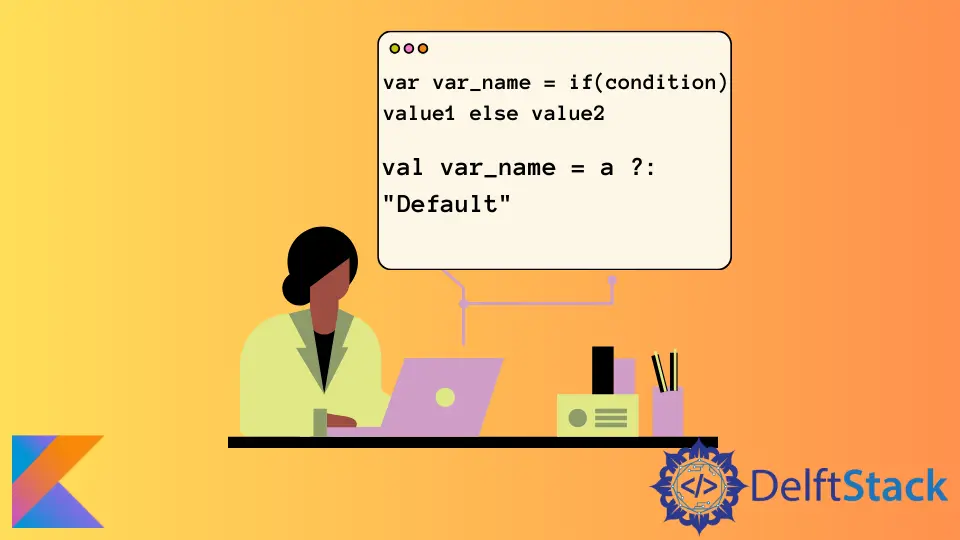
This tutorial teaches alternatives for Ternary Operators in Kotlin like if-else
expression, if-else-if
ladder, when
expression, and Elvis operator.
The Ternary Operator in Kotlin
Syntax:
int var_name = condition ? value if true : value if false
We can put a condition using <
, >
, ==
, etc., in a condition block that returns Boolean. If the condition evaluates to true, the value before :
is assigned, else the value after :
(colon) is assigned.
For example, let us take two numbers and compare them to print the smallest one using Java.
Using if-else
in Java:
public static void main(String[] args) {
int num1 = 82731, num2 = 32854;
int small = 0;
if (num1 > num2) {
small = num2;
} else
small = num1;
System.out.println(small);
}
Using Ternary Operator in Java:
public static void main(String[] args) {
int num1 = 82731, num2 = 32854;
int small = num1 > num2 ? num2 : num1;
System.out.println(small);
}
if_else
takes lines of code, but we can write it in a single line using the ternary operator. Thus, it makes the code shorter and easy to understand.
Use if-else
in Kotlin
The if-else
are not just statements but are also expressions in Kotlin. In other words, we can use if-else
blocks to check conditions and return values like functions or methods.
Syntax:
var var_name = if(condition) value1 else value2
var_name
is a variable. if
checks for condition, if it is true
it assigns value1
, else var_name
will be equal to value2
.
Example:
fun main(){
var num1 = 82731
var num2 = 32854
var small = if(num1>num2) num2 else num1
println(small)
}
Output:
32854
Here in the above example, if the if
condition is true, it returns num2
and assigns the small value as num2
. Else, small will be equal to num1
.
Use if-else-if
Ladder in Kotlin
We can also use the if-else-if
ladder as an expression to assign value to variables. It is the same as if-else
, but we can use it for multiple conditions.
Syntax:
var var_name = if(condition1) value1
else if(condition2) value2
.
.
.
else default_value
Here we can take multiple conditions, and for each condition, we can apply different values, else at the end of the ladder is compulsory.
Example:
fun main(){
println("Select your order")
println("a : Pizza \nb : French Fries \nc : Burger")
var ans = readLine()
var message = if(ans == "a") "Yummy! Your Pizza will be delivered soon"
else if(ans == "b") "Great Pick! Your French Fries will be there at your doorstep in 30 mins"
else if(ans == "c") "Your Burger is almost ready, Bon appetit!"
else "Invalid Order"
println(message)
}
Output:
Select your order
a : Pizza
b : French Fries
c : Burger
c
Your Burger is almost ready, Bon appetit!
We firstly show the menu to the user and take user preferences. As the user enters his/her choice, we store the message corresponding to the choice in the message
variable and then print the message.
Use when
Expression in Kotlin
We can also use when
as an expression and ternary operator.
Syntax:
var var_name = when(var2){
condition1 -> value1
condition2 -> value2
.
.
.
else -> value_default
}
We pass var2
as input when, if any conditions are satisfied, its value is assigned to the variable var_name
. If all the condition fails, the block is executed, and value_default
is assigned to var_name
.
Example:
fun main(){
print("Enter Percentage : ")
var percentage = Integer.valueOf(readLine())
var grade = when(percentage){
in 90..100 -> 'A'
in 80..90 -> 'B'
in 70..80 -> 'C'
in 60..70 -> 'D'
else -> 'E'
}
println("Grade : $grade")
}
Output:
Enter Percentage : 79
Grade : C
Use Elvis Operator (?:
) in Kotlin
Sometimes while cloning some values to another variable, we need to check if they are null
. This is made easier by Elvis Operator ?:
in Kotlin.
Instead of writing if-else
, we can write clean and compact code using the Elvis Operator.
Syntax:
val var_name = a ?: "Default"
Elvis operator checks if the given variable is null
. If it is null
, it assigns the new variable with the default value.
Example:
fun main(){
var first_name : String? = "Katie"
var last_name : String? = null
var full_name = first_name+" "+ (last_name ?: "")
System.out.println(full_name)
}
Output:
Katie
To generate ull_name
, we use first_name
and last_name
. Sometimes last_name
is null
, so we use Elvis Operator, and an empty string is used as default.
It only returns Katie
and not null
, as we can see in the result above.
Niyati is a Technical Content Writer and an engineering student. She has written more than 50 published articles on Data Structures, Algorithms, Git, DBMS, and Programming Languages like Python, C/C++, Java, CSS, HTML, KOTLIN, JavaScript, etc. that are very easy-to-understand and visualize.
LinkedIn