How to Suspend Function in Kotlin
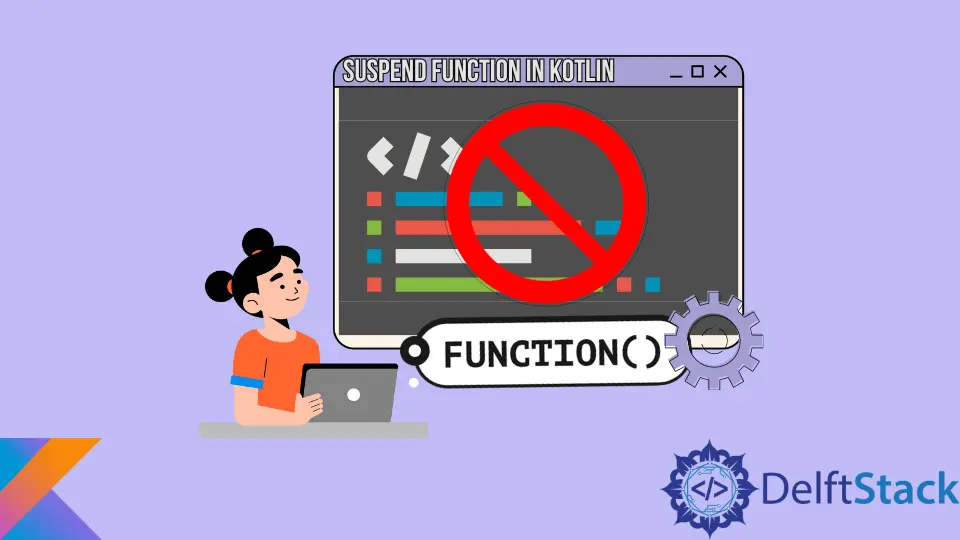
This article will introduce the suspend
function in Kotlin Coroutine and how to implement it.
the suspend
Function in Kotlin
Almost everything in Kotlin Coroutine revolves around the suspend
function. Coroutines are like lightweight threads that can run a block of code concurrently with the rest of the program.
Coroutines are not bound to any specific threads, so we can suspend them in one thread and resume them in another. The suspend
function can pause and continue at any given time.
It can allow a massive program to run and complete without interruption.
We can define a suspend
function by using the suspend
keyword before the function name while declaring it. But to execute a suspend
function, we need to invoke it either through another suspend
function or from a coroutine; otherwise, it will throw an error.
Although we define a suspend
function using the suspend
keyword, it is compiled as a standard one behind the screen. The compiled function takes an additional parameter Continuation<T>
.
Code:
suspend fun example(param: Int): Int {
// another running operation
}
The code above becomes,
fun example(param: Int, callback: Continuation<Int>): Int {
// another running operation
}
Here’s a simple example of the suspend
function:
import kotlinx.coroutines.*
fun main() = runBlocking {
launch { suspendExample() }
println("Hi")
}
suspend fun suspendExample() {
delay(2000L)
println("Welcome!")
}
Output:
Example of the suspend
Function in Kotlin
Let’s look at another suspended function in Kotlin to understand better how concurrent threads execute one after the other.
In this example, we will use 2 delay functions to display different print messages on the screen.
import kotlinx.coroutines.*
fun main() = runBlocking {
suspendExample()
println("Programming")
}
suspend fun suspendExample() = coroutineScope {
launch {
delay(1000L)
println("Kotlin")
}
launch {
delay(500L)
println("Welcome to")
}
println("Hello, ")
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn