Swift if Let Statement Equivalent in Kotlin
-
Compare Swift
if let
Statement and Kotlinlet
andrun
Functions -
Use the
let
andrun
Functions as Swiftif let
Statement Equivalent in Kotlin
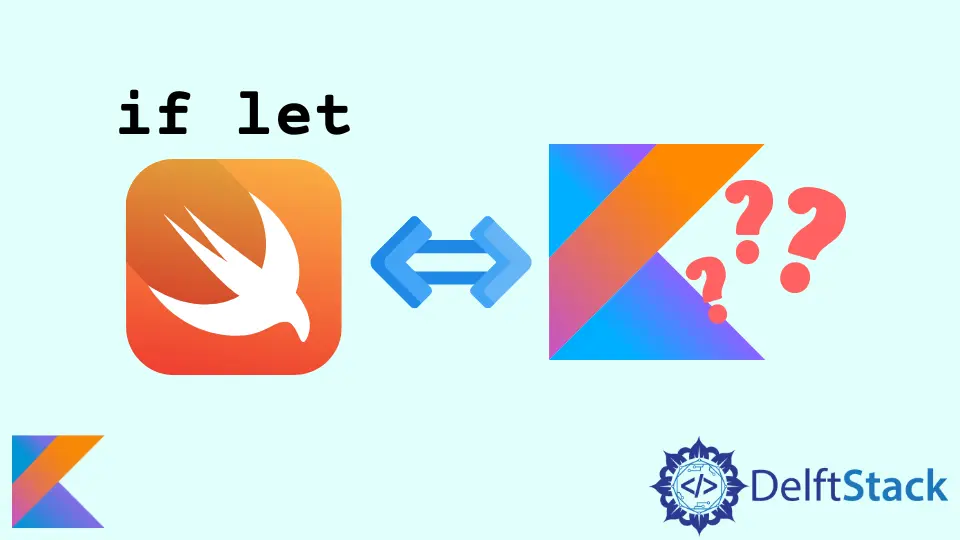
Swift if let
statements allow combining if
and null
checking statements into a single code block. It helps eliminate all the -nil pointer exceptional bugs by checking if the value of a variable or constant is null.
What if we want to use the same thing in Kotlin? In Kotlin, we can use let
and run
or the Elvis operator (? :
) as equivalent to the Swift if let
statement.
This article will demonstrate the let
and run
functions as Swift if let
statement equivalent in Kotlin.
Compare Swift if let
Statement and Kotlin let
and run
Functions
Here is how we can use the Swift if let
statement.
if let x = y.val {
} else {
}
Syntax for the let
and run
functions:
val x = y?.let {
// enter if y is not null.
} ?: run {
// enter if y is null.
}
In the syntax above, we need to use the run
block of code only if we have multiple lines of code after the Elvis operator. We can skip the run
block if there is only a single line of code post the Elvis operator.
Also, when using the Kotlin let
and run
, the compiler cannot access the variable from within the let
and run
blocks. Since the Kotlin Elvis operator is a nil-checker, the run
code will only execute if y
is null or if the let
block evaluates to null.
Use the let
and run
Functions as Swift if let
Statement Equivalent in Kotlin
Let us look at an example to understand how to implement Kotlin let
and run
functions in a program. This example will check the variable’s value.
The compiler will execute the let
block if it is null; otherwise, it will perform the run
block.
Example 1:
fun main(args: Array<String>) {
val y="Hello"
val x = y?.let {
// This code runs if y is not null
println("Code A");
} ?: run {
// This code runs if y is null
println("Code B");
}
}
Output:
The output runs the let
code since the value of y
is not null.
Now, let’s try by making the value of y
null.
Example 2:
fun main(args: Array<String>) {
val y= null
val x = y?.let {
// This code runs if y is not null
println("Code A");
} ?: run {
// This code runs if y is null
println("Code B");
}
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn