How to Use forEach in Kotlin
-
the
forEach
Loop in Kotlin -
Use
forEach
to Access List Items in Kotlin -
Use
forEach
to Perform Action on a List Item in Kotlin -
Use a Nested
forEach
Loop in Kotlin
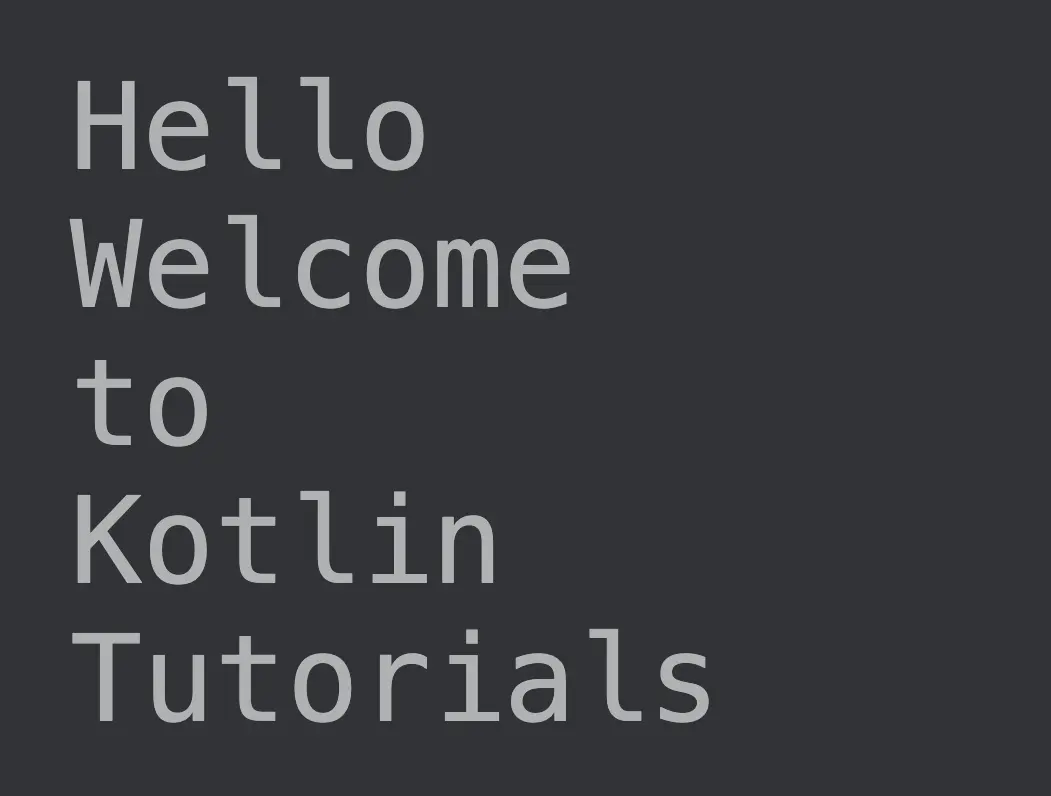
This article will introduce the concept and use of the forEach
loop in Kotlin.
the forEach
Loop in Kotlin
Kotlin forEach
is an iteration loop that allows you to access items of a collection or list and perform actions on each item. We can also do the same with the for
loop, but using multiple for
loops can quickly make your code messy.
On the other hand, forEach
makes your code more concise and easy to read. It can be more useful when using it as a functional operator.
We will use forEach
to access items on a list in this article.
Syntax:
list_name.forEach {
//statements
}
Use forEach
to Access List Items in Kotlin
We will start with a simple example where we access all the items on a list.
fun main(args: Array<String>) {
var myList = listOf("Hello", "Welcome", "to", "Kotlin", "Tutorials")
myList.forEach {
println(it)
}
}
Output:
Use forEach
to Perform Action on a List Item in Kotlin
Now, we know how to access the items. Let’s try to perform actions on them.
For this example, we will create an integer list. We will then use forEach
to iterate through each item and multiply it by 3.
fun main(args: Array<String>) {
var myList = listOf<Int>(3, 7, 11, 42, 50)
myList.forEach {
println(it*3)
}
}
Output:
Use a Nested forEach
Loop in Kotlin
We can also nest forEach
under one another. The example below demonstrates the use of nested forEach
in Kotlin.
fun main(args: Array<String>) {
var myList = listOf<Int>(1, 2)
myList.forEach {
println(it)
println()
myList.forEach {
println(it*3)
}
println()
}
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn