How to Use the Elvis Operator in Kotlin
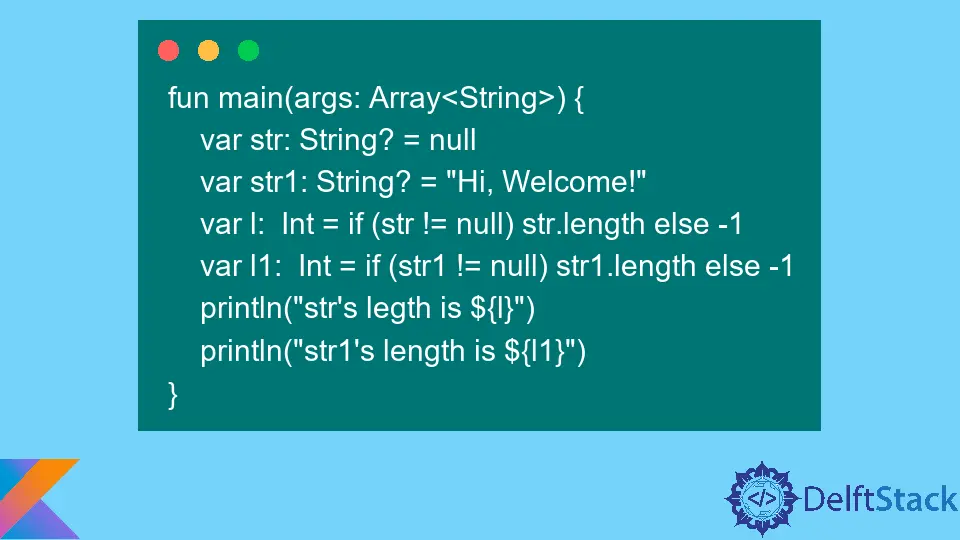
Do you remember Java’s ternary operator? Elvis operator in Kotlin is something similar to that.
The Elvis operator’s symbol is a question mark followed by a colon (?:
). It is a binary operator that will evaluate two expressions.
If the left expression is non-null, it will use the reference or value in that expression. But if the left expression has a null reference, it will execute the right expression.
The Elvis operator will evaluate the expression on the right side only if the expression on the left is null.
Use the Elvis Operator in Kotlin
Suppose you have a null value in a variable but want to use only a non-null value. You can do this using an if-else
statement.
fun main(args: Array<String>) {
var str: String? = null
var str1: String? = "Hi, Welcome!"
var l: Int = if (str != null) str.length else -1
var l1: Int = if (str1 != null) str1.length else -1
println("str's legth is ${l}")
println("str1's length is ${l1}")
}
Output:
The Elvis operator allows shortening the above code. So, we can use the Elvis operator instead of writing the entire if-else
statement.
Click here to check the demo of the example.
Here’s an example of the Elvis operator. We will try to execute the same results as in the above code, but this time using the Elvis operator.
fun main(args: Array<String>) {
var str: String? = null
var str1: String? = "Hi, Welcome!"
var l: Int = str ?.length ?: -1
var l1: Int = str1 ?.length ?: -1
println("str's legth is ${l}")
println("str1's length is ${l1}")
}
Output:
Click here to check the demo of the example.
Conclusion
We can also use the Elvis operator in Kotlin for null safety checks. Since throw
and return
are expressions, we can use them on the right-side of the Elvis operator for null safety checking.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn