Kotlin Data Class Inheritance: Extend a Data Class
-
Use the
data
Keyword to Define Class in Kotlin -
Requirements to Declare a
Data Class
in Kotlin -
Example of
Data Class
in Kotlin -
Extend
Data Class
in Kotlin -
Use the
Abstract Class
to Extend Data Class in Kotlin -
Use the
Interface
to Extend Data Class in Kotlin -
Use the
Open Classes
to Extend Data Class in Kotlin
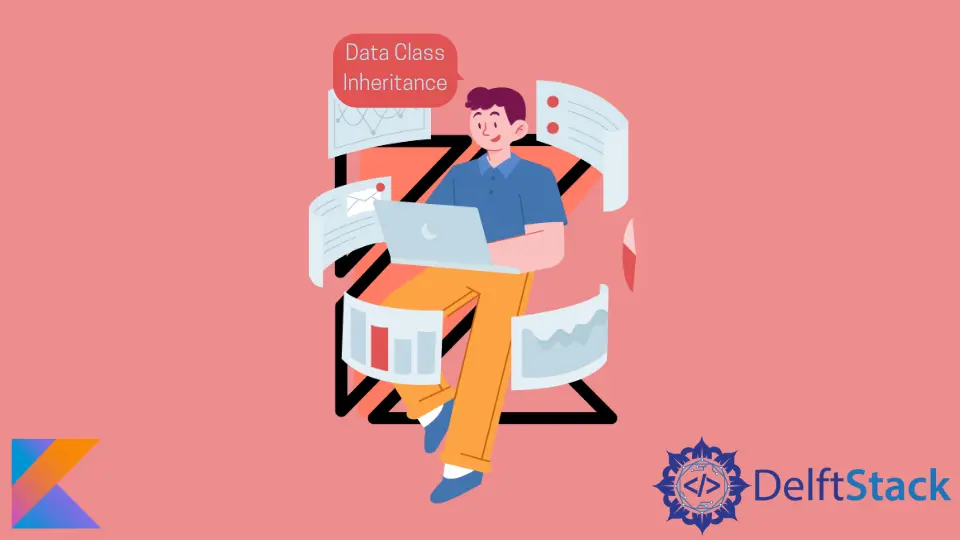
The data class
in Kotlin is the class that holds an object’s data.
This tutorial will show how to extend a data class
to leverage the concept of inheritance in Kotlin.
Use the data
Keyword to Define Class in Kotlin
Syntax:
data class Tutorials (var name: String, val year: Int)
Declaring a data class
in Kotlin automatically generates functions like equals()
, toString()
, and hashcode()
.
Requirements to Declare a Data Class
in Kotlin
- There should be one or more parameters in the
primary constructor
. - The parameters should be initialized as
var
orval
. - We cannot declare the class as
abstract
,open
,sealed
, orinner
.
Example of Data Class
in Kotlin
Use the main
function to declare variable properties in data class
.
data class Tutorial(val tutorial_name: String, val year: Int)
fun main(args: Array<String>) {
val tut = Tutorial("Kotlin", 2022)
println("Tutorial Name = ${tut.tutorial_name}")
println("Year = ${tut.year}")
}
Output:
Tutorial Name = Kotlin
Year = 2022
Extend Data Class
in Kotlin
Data classes are the replacements of POJOs in Java. Hence, it is natural to think that they would allow for inheritance in Java and Kotlin.
The inheritance of data classes in Kotlin doesn’t execute well. Hence, it is advised not to use inheritance by extending the data class in Kotlin.
But we can use abstract class
and interface
.
Use the Abstract Class
to Extend Data Class in Kotlin
The abstract class
can declare all the parameters as abstract in the parent class
and then override them in the child class
.
fun main(args: Array<String>) {
abstract class Tutorial {
abstract var year: Int
abstract var name: String
}
data class Book (
override var year: Int = 2022,
override var name: String = "Kotlin Tutorials",
var isbn: String
) : Tutorial()
}
The above code won’t throw any error as it extends the data class
. It will create a corresponding class and enable the data class to extend from it.
Use the Interface
to Extend Data Class in Kotlin
We can also extend a data class in Kotlin with the help of an interface
.
interface Base_Interface {
val item:String
}
interface Derived_Interface : Base_Interface {
val derived_item:String
}
fun main(args: Array<String>) {
data class B(override val item:String) : Base_Interface
data class D(override val derived_item:String,
private val b:Base_Interface) : Derived_Interface, Base_Interface by b
val b = B("Hello ")
val d = D("World!", b)
print(d.item) //from the base class
print(d.derived_item) //from the derived class
}
Use the Open Classes
to Extend Data Class in Kotlin
Another way to extend a data class
is by declaring all the parent class
properties as open
.
We can then use the same override method to override the properties in the sub-class
and extend the data class.
fun main(args: Array<String>) {
open class ParentClass {
var var1 = false
var var2: String? = null
}
data class ChildClass(
var var3: Long
) : ParentClass()
}
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn