How to Initialize Properties Using by Lazy and Lateinit in Kotlin
-
Initialize Properties Using
by lazy
in Kotlin -
Initialize Properties Using
lateinit
in Kotlin -
Difference Between
by lazy
andlateinit
in Kotlin
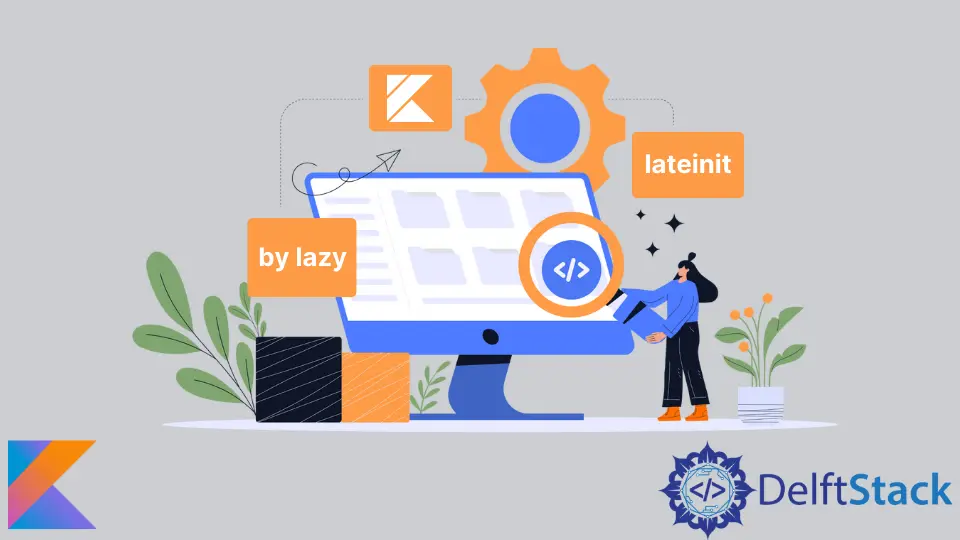
This article introduces the Kotlin by lazy()
and lateinit
that helps initialize a property. We will start by understanding what these property initializers do and then understand the difference between the two.
If we want to initialize a property outside the constructor or somewhere at the bottom of the class body, we have only two options: lazy initialization or lateinit
.
Initialize Properties Using by lazy
in Kotlin
The lazy initialization is done with the help of the lazy()
function. It takes a lambda as an input and returns an instance of lazy<t>
.
The instance serves the purpose of delegation required for implementing a lazy property. The evaluation of the lazy properties is synchronized by default.
The synchronization means that although the compiler computes the value in a single thread, all the threads will see the same value.
Kotlin allows preventing synchronization with the use of LazyThreadSafetyMode.PUBLICATION
as the lazy()
function’s parameter.
Example:
val lazyVal: String by lazy {
println("This is an")
"example of lazy initialization!"
}
fun main() {
println(lazyVal)
}
Output:
Initialize Properties Using lateinit
in Kotlin
Late initialization allows initializing non-null types properties outside the constructor while avoiding null checks.
Usually, non-null properties are initialized inside a constructor. But we may want otherwise in some situations like setting up a unit test method.
The lateinit
modifier helps solve such issues. We can use this initializer with var
or mutable properties.
Some points to remember about the lateinit
modifier are:
- We can use
lateinit
outside the primary constructor - We can use
lateinit
only for the properties that do not have customgetters()
andsetters()
. - The property’s type should be non-null and primitive
Example:
lateinit var student1:Student
fun main(args: Array<String>) {
student1 = Student("David Henry's",303)
print(student1.Name + " ID is " + student1.ID.toString())
}
data class Student(var Name:String, var ID:Int);
Output:
Difference Between by lazy
and lateinit
in Kotlin
Several significant differences exist between the by lazy
and lateinit
modifiers. Here are some of them:
by lazy
is used to initializeval
properties, andlateinit
is used forvar
properties.- The
lateinit
uses a backing field to store the result’s value, and theby lazy
uses a delegate object. - We cannot use
lateinit
with nullable properties or Java primitive types. - We can initialize
lateinit
from anywhere the compiler can see the object and support multiple initializations. On the other hand,by lazy
allows only a single initialization which is changeable only by overriding a property in a subclass. - The
by lazy
initialization is thread-safe by default, and thelateinit var
modifier’s initialization across multiple threads depends on the user’s code. - We can save, pass, and even use
by lazy
initializer’s instance for multiple properties. But there’s no additional runtime state inlateinit
.
When to Use by lazy
in Kotlin
We can use by lazy
when,
- we don’t want the variables to initialize unless we call or use them;
- we want to initialize a variable once and then use it throughout the code; and
- we are working with read-only properties.
When to Use lateinit
in Kotlin
We can use lateinit
when,
- we want to initialize a variable late;
- we want to initialize a variable before using it;
- we are working with
var
or mutable properties; and - the variables might change at a later stage.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn