How to Print to Console in Android Studio
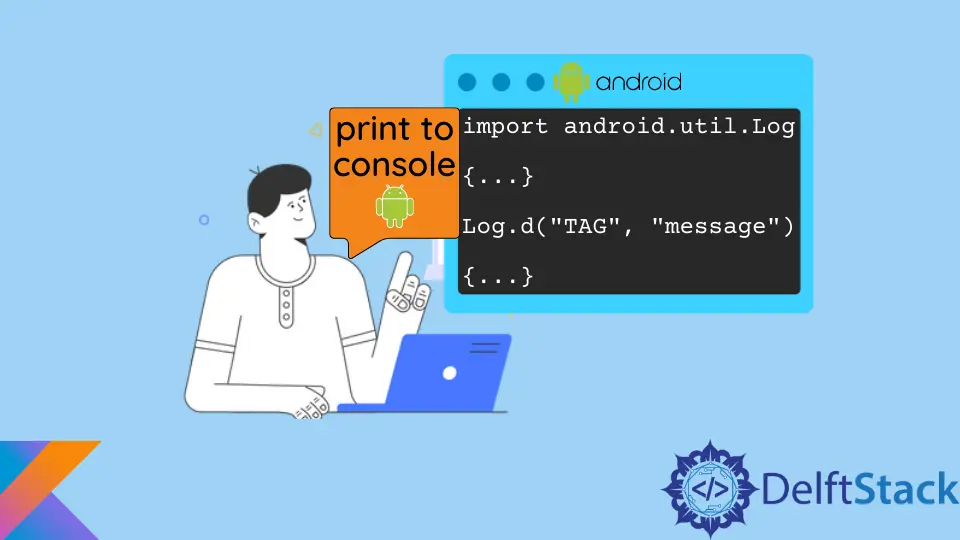
The console is the system’s monitor connected with an input device. We must display messages on the console to understand what the compiler is doing in the backend.
Suppose an error occurs while executing a code. How will we know what error has occurred unless we see it on the console?
Hence, printing to the console is of utmost importance.
There are different ways in different languages to print to console. We use printf()
in C, cout
in C++, System.out.println()
in Java and println()
in Kotlin.
But the Android Studio works a little differently. Using println()
in the Android Studio won’t display any message on the console in Kotlin.
So how can we print to console using Kotlin in Android Studio? This article will give you an answer for just that.
What is the Logcat Window
Before moving into how to print to console using Kotlin in Android Studio, we need to understand the Logcat Window. Well, Logcat Window is the Android Studio’s console.
Any message we print to the console is displayed on the Logcat Window.
Kotlin Print to Console in Android Studio
We can print to console using Kotlin in Android Studio with the help of the log
class. It is a predefined class that allows writing messages to the console.
We must import the log
class’ library android.util.Log
to print to the console using Kotlin.
Here’s the basic syntax to print to console.
import android.util.Log
{...}
Log.d("TAG", "message")
{...}
The letter d
in the above syntax denotes that the log message is for debugging the code. Similarly, the log
class has different letter indicators for different purposes, which are:
v
(Verbose
)d
(Debug
)i
(Information
)w
(Warning
)e
(Error
)a
(Assert
)
As we can see in the syntax, the log
class takes two arguments: "TAG"
and "message"
.
In the place of the "TAG"
, we need to write an indicator for the console command. And for the "message"
, we need to write a message we want to print on the console.
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn