How to Add Items to a List in Kotlin
-
Use the
+
Operator to Add Items to a List in Kotlin -
Use the
plus
Method to Add Items to a List in Kotlin -
Use the
Add()
Method to Add Items to a List in Kotlin
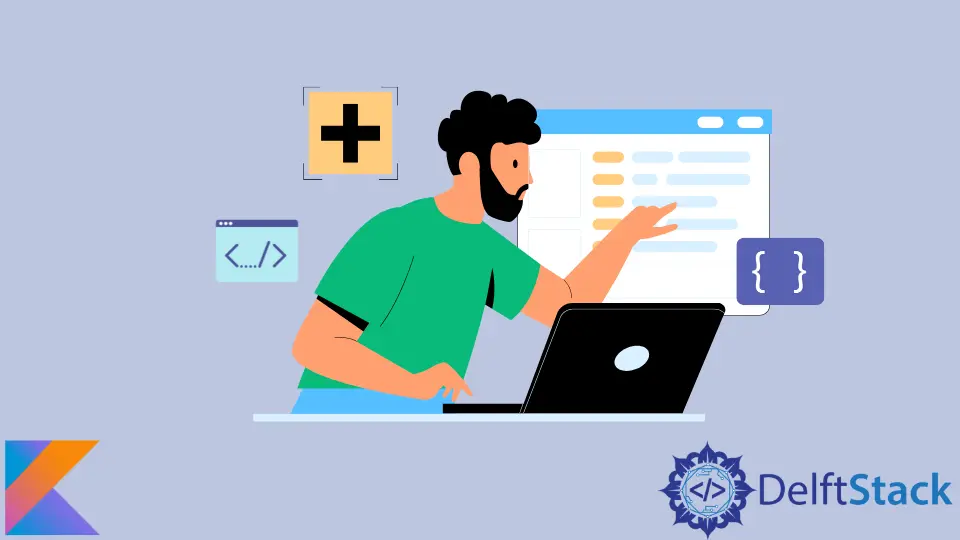
Suppose you have created a list in Kotlin. After making a list, you realize that you need to add more items. How will you do that?
This article lists different ways to add items to a list in Kotlin with some easy-to-digest examples.
Use the +
Operator to Add Items to a List in Kotlin
The first and easiest way to add more items to a list is using the +
operator. Using this operator returns the original list along with the newly added items.
We can use this operator to add both single elements and an entire collection. Below are a couple of examples where we use the +
operator to add items to a list.
The first example has a car list with the names of some popular cars. We will use the +
operator to add a single new item to the list.
Example:
fun main() {
val cars = listOf("Mercedes-Benz", "Porsche", "BMW")
val modified = cars + ("Ferrari")
println(modified)
}
Output:
We will use the same operator to add another list with three more names of car brands to our existing list.
Example:
fun main() {
val cars = listOf("Mercedes-Benz", "Porsche", "BMW")
val newList = listOf("Ferrari", "Lamborghini", "Bentley")
val modified = cars + newList
println(modified)
}
Output:
Use the plus
Method to Add Items to a List in Kotlin
Another way to add items to a list is using the plus
method. Like the +
operator, we can add a single item and a collection to a list.
As examples for this one, we will create a list of fruits and add new elements.
Example :
fun main() {
val fruits = listOf("Apple", "Mango", "Strawberry")
val modified = fruits + ("Pineapple")
println(modified)
}
Output:
Example :
fun main() {
val fruits = listOf("Apple", "Mango", "Strawberry")
val newList = listOf("Pineapple", "Orange", "Blueberries")
val modified = fruits.plus(newList)
println(modified)
}
Output:
Use the Add()
Method to Add Items to a List in Kotlin
Another method that allows adding items to a list is the add()
method.
However, the list needs to be mutable for this method to work. If we try using the add()
method with a standard list, it will throw an error.
Example:
fun main() {
val myList = listOf("One", "Two", "Three")
myList.add("Four")
println(myList)
}
Output:
As we can see, trying to add items to a standard list throws an error. Hence, we can add items only to a mutable list using the add()
method.
We can create a mutable list using mutableListOf<T>
. Here’s an example of adding the word Four
to the list.
Example:
fun main() {
val myList = mutableListOf("One", "Two", "Three")
myList.add("Four")
println(myList)
}
Output:
The catch with using the add()
method is that we can only add a single item to a list with this method. If we try to add a list, it will throw a type mismatch error.
Example:
fun main() {
val myList = mutableListOf("One", "Two", "Three")
val newList = mutableListOf("Four", "Five", "Six")
myList.add(newList)
println(myList)
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn