Equivalent of getClass() for KClass
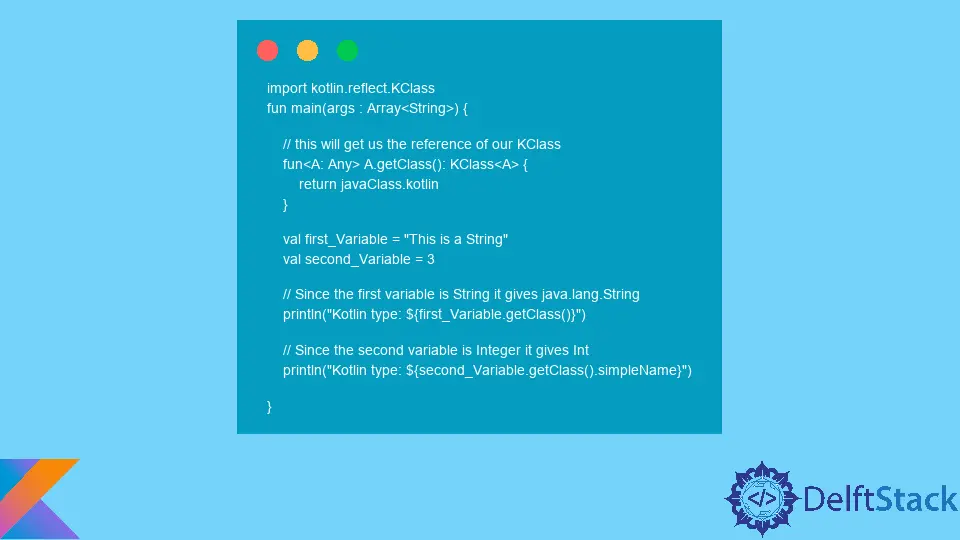
Java allows resolving a variable’s class with the getClass()
function and Kotlin allows that with the .javaClass()
function. The KClass type is Kotlin’s equivalent of Java’s java.lang.Class
type.
Let’s see how to get a reference of the KClass similarly.
the getClass()
Equivalent for KClass in Kotlin
The equivalent of something.getClass()
for KClass is something::class
. We can use this through the class reference syntax.
The class reference’s basic feature is to get a runtime reference to a KClass.
Syntax:
val v = Class_Name::class
Using ::class
is possible only in Kotlin 1.1 and onwards. To do this in Kotlin 1.0, we need to get the Java Class and convert it to Kotlin Class instance with the help of the .kotlin
property.
The syntax to get KClass instance in Kotlin 1.0 is:
Class_Name.javaClass.kotlin
We can also get the class object kotlin.reflect.KClass
by using KClass::class
. To get the current object’s class, we can use this::class
.
The following code block shows how to resolve a variable’s class in Kotlin.
Example Code:
import kotlin.reflect.KClass
fun main(args : Array<String>) {
// this will get us the reference of our KClass
fun<A: Any> A.getClass(): KClass<A> {
return javaClass.kotlin
}
val first_Variable = "This is a String"
val second_Variable = 3
// Since the first variable is String it gives java.lang.String
println("Kotlin type: ${first_Variable.getClass()}")
// Since the second variable is Integer it gives Int
println("Kotlin type: ${second_Variable.getClass().simpleName}")
}
Output:
Kailash Vaviya is a freelance writer who started writing in 2019 and has never stopped since then as he fell in love with it. He has a soft corner for technology and likes to read, learn, and write about it. His content is focused on providing information to help build a brand presence and gain engagement.
LinkedIn