How to Extend a Class Having Multiple Constructors in Kotlin
- Understanding Constructors in Kotlin
- Extending a Class with Multiple Constructors
- Best Practices for Using Constructors in Kotlin
- Conclusion
- FAQ
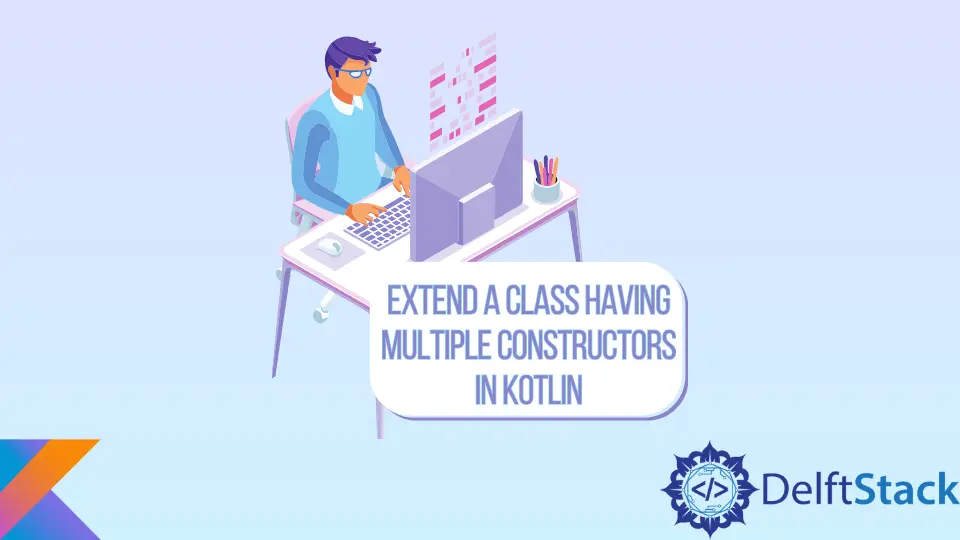
Kotlin, a modern programming language, offers a powerful and flexible way to create classes. One of its exciting features is the ability to extend classes that have multiple constructors.
This tutorial will guide you through the process of using secondary constructors to achieve this. You’ll learn how to define a base class with multiple constructors and then extend it with a derived class. By the end of this article, you’ll have a solid understanding of how to work with constructors in Kotlin, enabling you to write cleaner, more efficient code. Whether you’re a beginner or an experienced developer, this guide will enhance your Kotlin skills and help you make the most of class extensions.
Understanding Constructors in Kotlin
In Kotlin, classes can have primary and secondary constructors. The primary constructor is defined in the class header, while secondary constructors are defined within the class body. This flexibility allows developers to create instances of a class in various ways, depending on the parameters provided.
Primary Constructor
The primary constructor is a concise way to initialize class properties. It’s declared in the class header and can take parameters directly. For instance:
class Person(val name: String, var age: Int) {
init {
println("Person created: Name: $name, Age: $age")
}
}
Output:
Person created: Name: John, Age: 30
In this example, the Person
class has a primary constructor that initializes the name
and age
properties. The init
block runs immediately after the primary constructor, allowing for additional initialization logic.
Secondary Constructors
Secondary constructors provide an alternative way to create instances of a class. They are useful when you want to offer different ways to initialize a class. Here’s how you can define a secondary constructor:
class Person(val name: String) {
var age: Int = 0
constructor(name: String, age: Int) : this(name) {
this.age = age
println("Person created: Name: $name, Age: $age")
}
}
Output:
Person created: Name: John, Age: 30
In this case, the Person
class has a secondary constructor that takes both name
and age
. It calls the primary constructor using : this(name)
and then sets the age
property.
Extending a Class with Multiple Constructors
Now that you understand how to create classes with multiple constructors, let’s explore how to extend a class that has them. When you extend a class in Kotlin, you can call its constructors from the derived class. This allows you to build upon the functionality of the base class.
Example of Class Extension
Consider a base class Employee
with multiple constructors:
open class Employee(val name: String) {
var age: Int = 0
constructor(name: String, age: Int) : this(name) {
this.age = age
println("Employee created: Name: $name, Age: $age")
}
}
Output:
Employee created: Name: John, Age: 30
Now, let’s create a derived class Manager
that extends Employee
:
class Manager(name: String) : Employee(name) {
var department: String = ""
constructor(name: String, age: Int, department: String) : this(name) {
this.age = age
this.department = department
println("Manager created: Name: $name, Age: $age, Department: $department")
}
}
Output:
Manager created: Name: John, Age: 30, Department: Sales
In this example, the Manager
class extends the Employee
class. It has its own secondary constructor that initializes the department
property while also calling the base class constructor.
Best Practices for Using Constructors in Kotlin
When working with constructors in Kotlin, there are a few best practices to keep in mind to ensure your code remains clean and maintainable.
Use Primary Constructors When Possible
Whenever you can, prefer using primary constructors for simple initialization. They help keep your code concise and easy to read. For example, if your class has only a few properties, a primary constructor is usually sufficient.
Keep Secondary Constructors Simple
If you need to use secondary constructors, try to keep them straightforward. They should serve a clear purpose, such as providing alternative initialization options. Avoid adding too much logic within the secondary constructors, as this can lead to confusion.
Leverage Default Parameter Values
Kotlin supports default parameter values, which can reduce the need for multiple constructors. Instead of defining several constructors for different combinations of parameters, you can provide default values in the primary constructor. For instance:
class Employee(val name: String, var age: Int = 30)
This allows you to create an Employee
instance with just the name, while still providing an age if necessary.
Conclusion
Extending a class with multiple constructors in Kotlin is a straightforward process that enhances code reusability and flexibility. By leveraging primary and secondary constructors, you can create robust class hierarchies that cater to various initialization scenarios. Remember to keep your constructors clean and utilize default parameter values when possible. With these techniques in your toolkit, you’ll be well-equipped to tackle more complex programming challenges in Kotlin. Happy coding!
FAQ
- What is the difference between primary and secondary constructors in Kotlin?
The primary constructor is defined in the class header and initializes properties directly, while secondary constructors are defined within the class body and provide alternative ways to create instances.
-
Can a class have multiple primary constructors in Kotlin?
No, a class can have only one primary constructor. However, it can have multiple secondary constructors. -
How do I call a secondary constructor from a derived class?
You can call a secondary constructor from a derived class using the: this(...)
syntax, similar to how you call the primary constructor. -
What are default parameter values in Kotlin?
Default parameter values allow you to specify a default value for a parameter, enabling you to omit that parameter when calling the constructor. -
Is it possible to have a constructor without parameters in Kotlin?
Yes, you can define a constructor without parameters in Kotlin, which is often used for classes that do not require any initial values.
I am Fariba Laiq from Pakistan. An android app developer, technical content writer, and coding instructor. Writing has always been one of my passions. I love to learn, implement and convey my knowledge to others.
LinkedIn