How to Toggle Class in jQuery
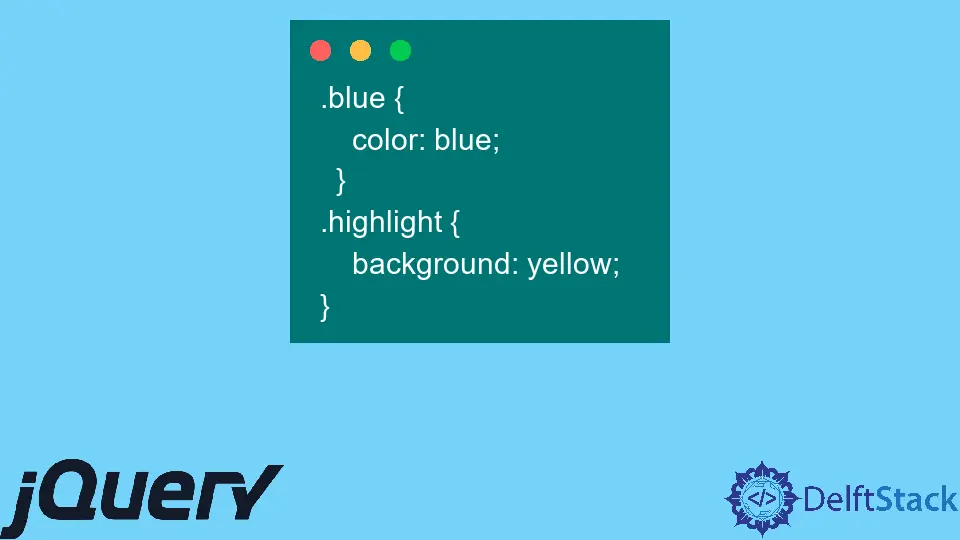
Today’s post will teach about the toggle
class in jQuery.
Toggle Class in jQuery
jQuery provides a built-in class toggle
method. This event adds or removes one or more classes from each element in the set of matching elements based on the existence of the class or the value of the status argument.
Syntax:
.toggleClass(className).toggleClass(classNames)
className
is one or more classes (separated by spaces) to toggle for each element in the matched set.classNames
is an array of classes that toggle for each element in the matching set.
The toggle
method takes one or more classes as parameters. If an element in the matching element set already has the class in the first version, it is removed.
If an element doesn’t have the class, it will be added. We can apply .toggleClass()
to a simple <div>
for example.
The second model of .toggleClass()
uses the second parameter to determine whether or not to add or eliminate the class. If this parameter’s value is true
, the class is added; if false
, the class is eliminated.
If no arguments are exceeded to .toggleClass()
toggles all classes of the element when .toggleClass()
is invoked as for the first time.
Let’s understand it with the following example.
<p class="blue">Click on me to change color</p>
.blue {
color: blue;
}
.highlight {
background: yellow;
}
$('p').click(function() {
$(this).toggleClass('highlight');
});
The above example has defined a paragraph tag with a blue
class. Once the user has clicked on the paragraph, it will apply .toggleClass("highlight")
to the paragraph.
Run the above code in any browser that supports jQuery; it will display the result below.
Output:
Before toggle
:
After toggle
:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn