How to Get Value of Select OnChange in jQuery
- Understanding the jQuery Select OnChange Event
- Enhancing Functionality with Multiple Selects
- Using AJAX to Update Content Based on Selection
- Conclusion
- FAQ
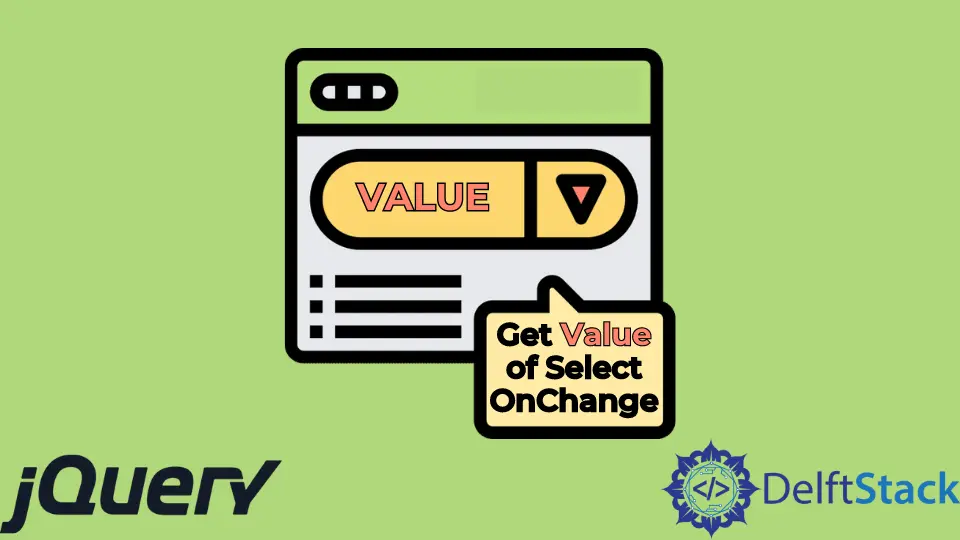
In today’s post, we’ll learn about getting the changed values of the select in jQuery. This is a common requirement in web development, especially when you want to create dynamic interfaces that respond to user input. When a user selects an option from a dropdown, you may want to capture that value for further processing, such as updating other elements on the page or sending it to a server. jQuery makes this process straightforward with its powerful event handling capabilities. By the end of this article, you’ll be equipped with the knowledge to effectively use jQuery to capture the value of a select element when it changes.
Understanding the jQuery Select OnChange Event
The change
event in jQuery is triggered when the value of a select element changes. This event can be used to execute a function that captures the new value and performs any necessary actions. To set this up, you’ll need to include jQuery in your project. Here’s a simple example of how to get started.
Basic Example of Select OnChange
Here’s a simple HTML structure with a select element:
<select id="mySelect">
<option value="Option1">Option 1</option>
<option value="Option2">Option 2</option>
<option value="Option3">Option 3</option>
</select>
<p id="selectedValue"></p>
Now, let’s add jQuery to capture the selected value when the user changes the selection:
$(document).ready(function() {
$('#mySelect').change(function() {
var selectedValue = $(this).val();
$('#selectedValue').text('You selected: ' + selectedValue);
});
});
When a user selects a different option, this code captures the new value and displays it in a paragraph below the select box.
Output:
You selected: Option2
This example is straightforward. We first select the dropdown using its ID and then listen for the change
event. When the event occurs, we retrieve the selected value using the val()
method and display it in a paragraph element. This is a great way to provide immediate feedback to the user.
Enhancing Functionality with Multiple Selects
In many applications, you might have multiple select elements that require similar handling. jQuery allows us to scale our code easily. Let’s consider a scenario where we have two select elements, and we want to display the selected values from both.
Example with Multiple Selects
Here’s an example HTML structure with two select elements:
<select id="firstSelect">
<option value="First1">First Option 1</option>
<option value="First2">First Option 2</option>
</select>
<select id="secondSelect">
<option value="Second1">Second Option 1</option>
<option value="Second2">Second Option 2</option>
</select>
<p id="combinedValue"></p>
Now, let’s write the jQuery code to handle changes in both selects:
$(document).ready(function() {
$('#firstSelect, #secondSelect').change(function() {
var firstValue = $('#firstSelect').val();
var secondValue = $('#secondSelect').val();
$('#combinedValue').text('First: ' + firstValue + ', Second: ' + secondValue);
});
});
With this code, when either select changes, it updates a paragraph with the selected values from both dropdowns.
Output:
First: First2, Second: Second1
This code snippet demonstrates how you can manage multiple select elements efficiently. By using a single change event listener for both selects, we reduce redundancy and keep our code clean. This is particularly useful in complex forms where users need to select multiple options.
Using AJAX to Update Content Based on Selection
Sometimes, you may want to fetch data from a server based on the selected value and update the content dynamically. jQuery’s AJAX capabilities make this easy. Let’s see how to implement this.
Example with AJAX
Assuming you have a server endpoint that returns data based on the selected value, here’s how you can set it up:
<select id="dataSelect">
<option value="Data1">Data 1</option>
<option value="Data2">Data 2</option>
</select>
<div id="dataDisplay"></div>
Now, let’s add the jQuery code to fetch and display data when the selection changes:
$(document).ready(function() {
$('#dataSelect').change(function() {
var selectedData = $(this).val();
$.ajax({
url: '/getData',
type: 'GET',
data: { data: selectedData },
success: function(response) {
$('#dataDisplay').html(response);
}
});
});
});
In this example, when the user selects an option, an AJAX GET request is sent to the server with the selected value. The server responds with data, which is then displayed in a div
.
Output:
Data for Data1
This method allows for a more interactive user experience. Users can select an option, and based on that selection, relevant information is fetched and displayed without needing to refresh the page. This is particularly useful in applications that require real-time data updates.
Conclusion
Capturing the value of a select element on change using jQuery is a fundamental skill for web developers. Whether you’re working with a single dropdown or multiple selects, jQuery provides the tools necessary to make your web applications dynamic and responsive. From providing immediate feedback to users to fetching data via AJAX, the possibilities are endless. With the techniques discussed in this article, you can enhance user experience and create interactive web applications that respond effectively to user input.
FAQ
-
How do I include jQuery in my project?
You can include jQuery in your project by adding a script tag in your HTML file that links to the jQuery CDN. -
Can I use jQuery with other JavaScript libraries?
Yes, jQuery can be used alongside other JavaScript libraries, but you may need to ensure there are no conflicts. -
What if I want to execute multiple functions on change?
You can call multiple functions within the change event handler, just separate them with a semicolon. -
Is it possible to capture the previous value of the select?
Yes, you can store the previous value in a variable before updating it in the change event handler. -
Can I use jQuery with frameworks like React or Angular?
While it’s possible, it’s generally not recommended to mix jQuery with these frameworks since they manage the DOM differently.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn