How to Trigger Click in jQuery
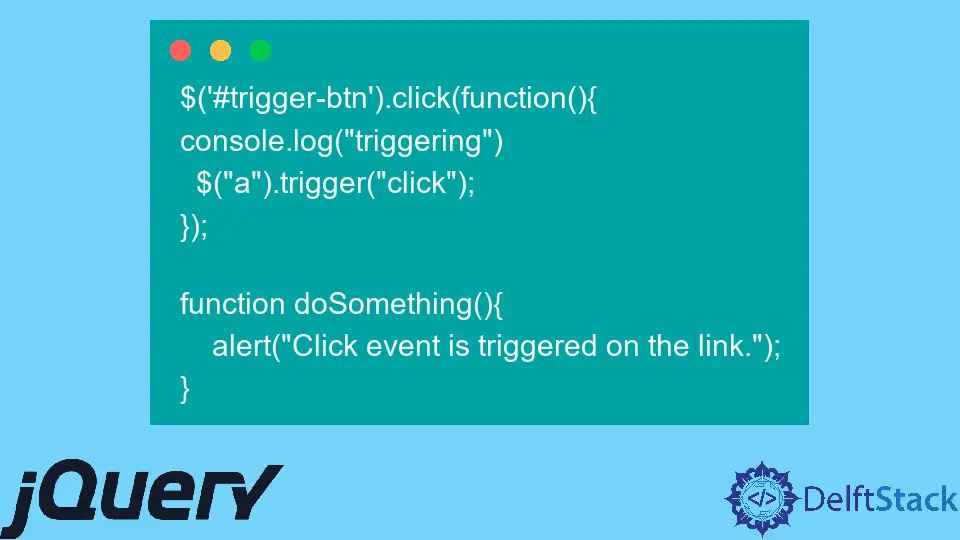
Today’s post will teach about jQuery’s trigger
click event.
Trigger Click Event in jQuery
jQuery provides a trigger
method that runs any handlers and behaviors attached to matching items for the specified event type.
Syntax:
.trigger(eventType[, extraParameters])
eventType
is a string containing a JavaScript event type, such as a click or submit.extraParameters
are additional parameters to pass to the event handler.
Any event handlers associated with .on()
or one of its shortcut techniques are precipitated while the corresponding event occurs. However, they can be triggered manually using the .trigger()
method.
A call to .trigger()
executes the handlers inside the identical order they might be if the event had been triggered certainly through the user.
As of jQuery 1.3, .trigger()
events appear in the DOM tree; an event handler can prevent the bubbling by returning false
from the handler or calling the .stopPropagation()
approach on the event object exceeded into the event. Despite .trigger()
simulating an event activation whole with a synthesized event object, it does now not perfectly replicate a certainly-going on the event.
The event object is constantly passed as the first parameter to an event handler. An array of arguments also can be handed to the .trigger()
call, and those parameters might be surpassed along to the handler as properly following the event object.
As of jQuery 1.6.2, a single string or numeric argument can be passed without being enclosed in an array.
The .trigger()
technique can be used in jQuery collections that wrap simple JavaScript objects similar to a Pub/Sub
mechanism; any given event handler for the object could be invoked when the event is raised.
Let’s understand it with the following example.
<a onclick="doSomething()">Google</a>
<button type="button" id="trigger-btn">Trigger</button>
$('#trigger-btn').click(function() {
console.log('triggering')
$('a').trigger('click');
});
function doSomething() {
alert('Click event is triggered on the link.');
}
In the example above, we have defined the click
function that notifies the user. You can pass the href
attribute with a specific link.
Let’s take an example where another element should trigger this click
. In this example, we have defined the trigger
button that triggers the click
.
Attempt to run the above code snippet in any browser that supports jQuery; it will display the result below.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn