How to Set Selected Option in jQuery
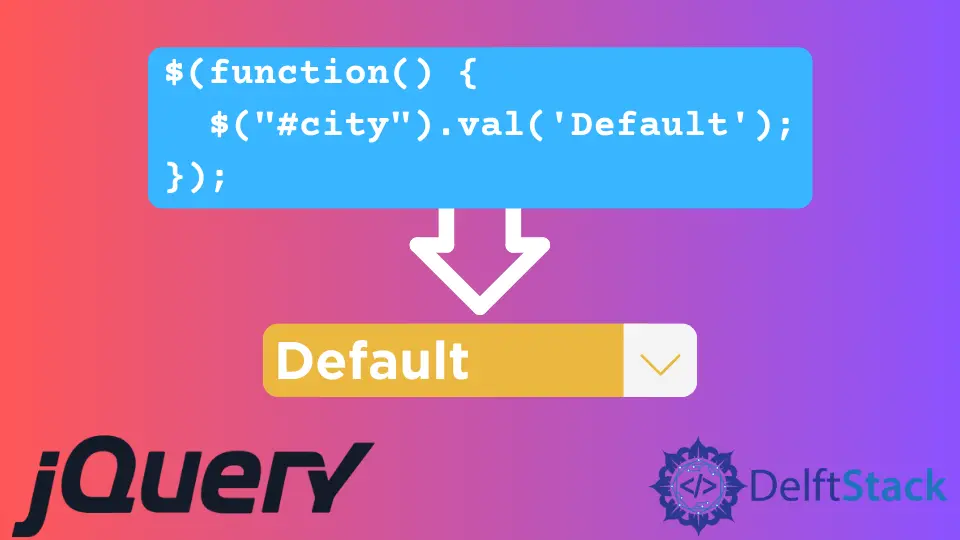
Today’s post will teach setting the default value of select
in jQuery.
Set Selected Option in jQuery
This method is typically used to set form field values. It sets the value of each item in the set of matching items.
A text string, number, or array of strings corresponding to the value of each matching item to be designated as selected/checked. val()
allows users to pass an array of element values.
It is useful when working on a jQuery object that contains elements like <input type="checkbox">
, <input type="radio">
and <option>
within <select>
.
In this case, entries and options with a value that matches one of the array elements are checked or selected, while those whose value does not match any of the array elements are checked or unchecked.
In the case of <input type="radio">
that is part of a radio group and <select>
, any previously selected item will be deselected. Setting values with this method does not cause the change event to fire.
For this reason, the corresponding event handlers are not executed. You have to call.trigger("change")
after setting the value if you want to run them.
The .val()
method allows you to set the value by passing a function. As of jQuery 1.4, two arguments are passed to the function: the current element’s index and its current value.
You can find more information in this documentation.
Let’s understand it with the following example.
<select id="city">
<option value="mumbai">Mumbai</option>
<option value="goa">Goa</option>
<option value="delhi">Delhi</option>
</select>
$(function() {
$('#city').val('goa');
});
In the above example, we have defined a city
dropdown using select
. The default value for the city dropdown is goa
.
Run the above code snippet in any browser that supports jQuery; it will show the below result.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn