How to Set Checkbox Checked Using jQuery
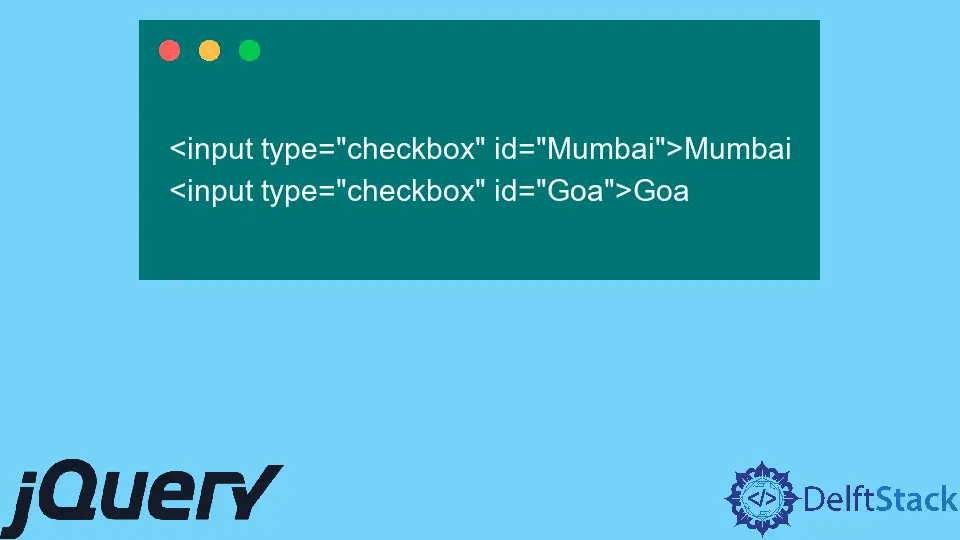
In this post, we’ll learn about setting checkboxes checked in jQuery.
Set Checkbox Checked in jQuery
This jQuery method accepts the attribute’s name, whose value should be updated and associated with the element. jQuery’s .attr()
method is used to set one or more attributes for the set of matched items/elements.
Syntax:
attr(attributeName, value)
attributeName
is the first input parameter of the typestring
that indicates the name of the attribute whose value is to be set.value
is the second input parameter of typestring
ornull
ornumber
that indicates the value set for the selected attribute. If the value isnull
, the specified attribute will be removed, similar to.removeAttr()
.
The .attr()
technique is handy to set the value of attributes - specifically while putting more than one attribute or using values returned by a function. The .attr()
approach reduces inconsistencies.
You can find more information about the .attr()
of jQuery in the documentation for .attr()
.
Let’s have an example.
Code - HTML:
<input type="checkbox" id="Mumbai">Mumbai
<input type="checkbox" id="Goa">Goa
Code - JavaScript + jQuery:
$('#Goa').attr('checked', 'checked');
We described two checkboxes, each assigned a different id. You can access the checked attribute of the checkbox using the attr()
method.
This method allows you to update the attribute of the checkbox to be set as the default value. Run the example code above in any browser that supports jQuery to display the following result.
Output:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn