How to Set a New Value for the Attribute Using jQuery
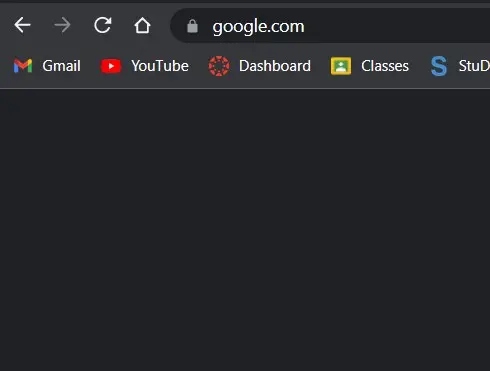
This article will demonstrate how to set a new value for the attribute using jQuery.
Set a New Value for the Attribute Using jQuery
The attr()
operation sets or returns the attributes and values of the components that have been chosen. When used to replace the attribute value, this function delivers the value of the FIRST matched element.
This method sets one or more attribute/value pairs for the collection of matched items when used to set attribute values.
Syntax:
$(selector).attr(attribute_name, new_value)
The attr()
method used here is for setting a new value for the attribute, which takes the parameter such as attribute name and value.
Example:
<html>
<head>
<title>Set New Attribute</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<script>
$(document).ready(function () {
$("button").click(function () {
$("#naya").attr("href", "https://www.youtube.com/");
});
});
</script>
</head>
<body>
<p><a href="https://www.google.com/" id="naya">ATT_Link</a></p>
<button>new value</button>
<p>
first, you can view the link and then click on button new value. it will
change the attribute value.
</p>
</body>
</html>
The above example shows that the attribute’s name is href
, and its new value is a link to youtube. The selector is a tag whose new value is set.
The selector used here is naya
, i.e., the id of the angular label with href
. When you run this code in the browser, you will see the following:
Here, you can see one link and one button. When you first click on the link, it will open google.com
, as mentioned in the code.
After that, when you click on the button named new value
, you will see the initial value of the attribute href
is changed to youtube.com
. After clicking on the button, it will trigger the click
function and then changes the attribute value with the help of the attr()
method.
Take a look at the screenshot below:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn