jQuery ID Selector
- What is the jQuery ID Selector?
- Basic Usage of the ID Selector
- Chaining Methods with the ID Selector
- Handling Events with the ID Selector
- Conclusion
- FAQ
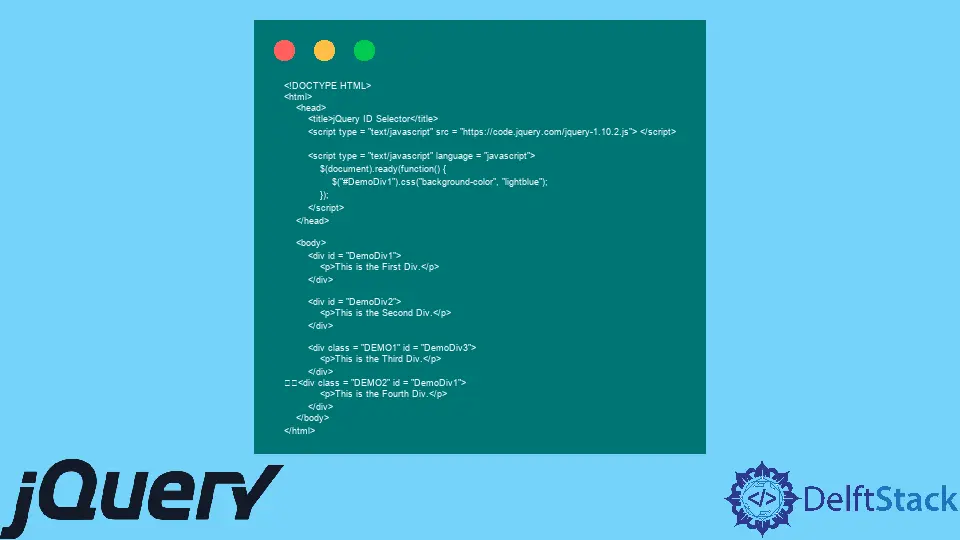
When it comes to selecting elements in your HTML document, jQuery offers a powerful toolset that simplifies the process. Among these tools, the ID selector is one of the most commonly used methods. It allows developers to target specific elements quickly and efficiently, making it an essential part of any jQuery toolkit.
In this tutorial, we’ll explore how to use the ID selector in jQuery, discussing its syntax, advantages, and providing practical examples. Whether you’re a beginner or an experienced developer, understanding how to effectively use the ID selector can significantly enhance your web development workflow.
What is the jQuery ID Selector?
The jQuery ID selector uses the syntax $("#id")
to select an HTML element with a specific ID. This is one of the fastest ways to access elements because IDs are unique within a document. For example, if you have an HTML element like <div id="myElement"></div>
, you can easily manipulate it using jQuery.
Advantages of Using the ID Selector
- Speed: Since IDs are unique, jQuery can quickly find the element without searching through the entire DOM.
- Simplicity: The syntax is straightforward, making it easy for developers to read and write.
- Direct Manipulation: Once selected, you can easily apply various jQuery methods to modify the element’s attributes, styles, or content.
Basic Usage of the ID Selector
To illustrate how to use the ID selector in jQuery, let’s consider a simple example. Imagine you have a button that, when clicked, changes the text of a specific paragraph.
Example Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>jQuery ID Selector Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#changeText").click(function() {
$("#myParagraph").text("Text has been changed!");
});
});
</script>
</head>
<body>
<p id="myParagraph">This is the original text.</p>
<button id="changeText">Change Text</button>
</body>
</html>
In this example, we have a paragraph with the ID myParagraph
and a button with the ID changeText
. When the button is clicked, the text of the paragraph changes to “Text has been changed!” The jQuery code uses the ID selector to bind a click event to the button and to change the text of the paragraph.
Chaining Methods with the ID Selector
One of the powerful features of jQuery is its ability to chain methods. This allows you to perform multiple actions on the same element in a single line of code. Let’s enhance our previous example by changing both the text and the color of the paragraph when the button is clicked.
Example Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>jQuery ID Selector Chain Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#changeText").click(function() {
$("#myParagraph").text("Text has been changed!").css("color", "red");
});
});
</script>
</head>
<body>
<p id="myParagraph">This is the original text.</p>
<button id="changeText">Change Text and Color</button>
</body>
</html>
In this example, we added a .css()
method to change the text color to red. By chaining methods, we keep our code clean and efficient. This not only improves readability but also enhances performance since jQuery minimizes the number of times it needs to traverse the DOM.
Handling Events with the ID Selector
Another important aspect of using the jQuery ID selector is event handling. You can easily attach various event handlers to elements selected by their ID. Let’s take our example a step further by adding a second button that resets the text when clicked.
Example Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>jQuery ID Selector Event Handling Example</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<script>
$(document).ready(function() {
$("#changeText").click(function() {
$("#myParagraph").text("Text has been changed!").css("color", "red");
});
$("#resetText").click(function() {
$("#myParagraph").text("This is the original text.").css("color", "black");
});
});
</script>
</head>
<body>
<p id="myParagraph">This is the original text.</p>
<button id="changeText">Change Text and Color</button>
<button id="resetText">Reset Text</button>
</body>
</html>
In this example, we introduced a second button with the ID resetText
. When clicked, it resets the paragraph text back to its original state and changes the color back to black. This demonstrates how versatile the jQuery ID selector can be for managing user interactions on a webpage.
Conclusion
The jQuery ID selector is a vital tool in web development that allows for quick and efficient manipulation of HTML elements. Its unique ability to target specific elements by their ID makes it an essential part of any jQuery script. From changing text and styles to handling events, the ID selector can streamline your coding process and enhance user experience. By mastering the ID selector, you can take your web development skills to the next level.
FAQ
- What is the jQuery ID selector?
The jQuery ID selector is a method used to select an HTML element by its unique ID, using the syntax $("#id").
-
Why should I use the ID selector?
The ID selector is fast and efficient because IDs are unique in a document, allowing for quick access and manipulation of elements. -
Can I chain methods with the ID selector?
Yes, jQuery allows you to chain multiple methods together, making your code cleaner and more efficient. -
How do I handle events using the ID selector?
You can attach event handlers to elements selected by their ID, allowing you to respond to user interactions easily. -
Is the ID selector case-sensitive?
Yes, the ID selector is case-sensitive, meaning that#myElement
and#myelement
would refer to different elements.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook