How to Select by Name in jQuery
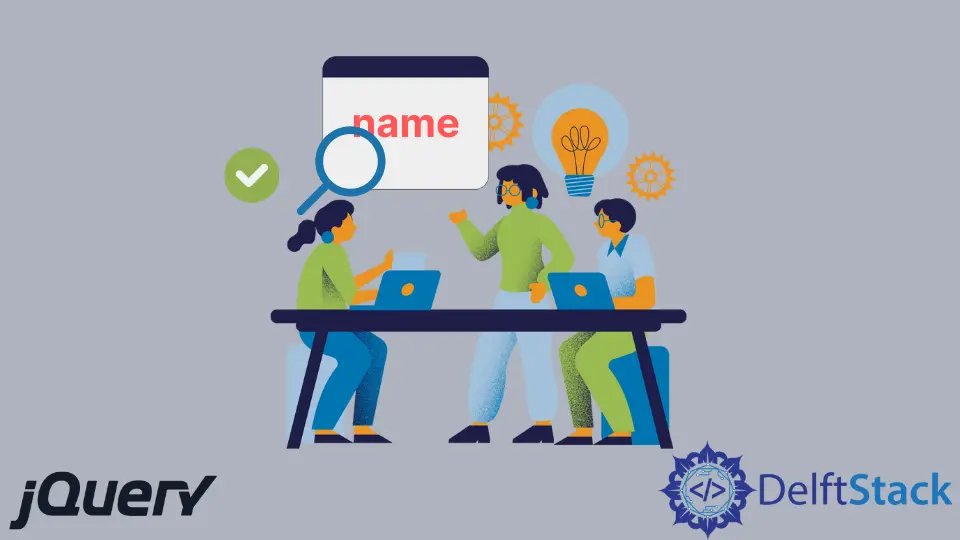
Today’s post will teach selecting elements using the name
attributes in jQuery.
Select by Name in jQuery
The CSS specification allows elements to be identified based on their attributes. While some older browsers don’t support it for document design purposes, jQuery allows you to use it regardless of your browser.
When using any of the attribute selectors below, you must be aware of attributes with multiple values separated by spaces because these selectors see the attribute values as a single string.
The following are the ways to select the attribute using the name
property.
- Attribute Prefix Selector
[name|="value"]
- It selects items where the specified attribute is equal to or begins with a specified string followed by a hyphen. - Attribute Selector
[name*="value"]
- It selects items with the specified attribute with a value containing a specified substring. - Attribute Word Selector
[name~="value"]
- It selects items with the specified attribute with a value containing a specific word, separated by spaces. - Attribute Ends With Selector
[name$="value"]
- It selects items with the specified attribute with a value ending in exactly one specific string. The comparison is case-sensitive. - Attribute Equals Selector
[name="value"]
- It selects items with the specified attribute with a value exactly equal to a specified value. - Attribute Starts With Selector
[name^="value"]
- It selects items with the specified attribute with a value that starts with exactly one specified string.
Let’s understand it with the following example.
<button name="btn-0" value="hello world">Hello World!</button>
console.log($('[name="btn-0"]').val());
console.log($('[name^="btn"]').val());
console.log($('[name$="-0"]').val());
console.log($('[name*="tn-0"]').val());
In the above example, we have defined one button with the name btn-0
and the value hello world
. We can select the button in 4 ways.
The first one selects the button using the exact name of the button. The second one selects the button whose name starts with btn
.
The third one selects the button whose value ends with -0
. And the last one selects the button whose name attribute contains the tn-0
.
Run the above code snippet in any browser that supports jQuery; it will show the below result.
Output:
"hello world"
"hello world"
"hello world"
"hello world"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn