The resize() Method in jQuery
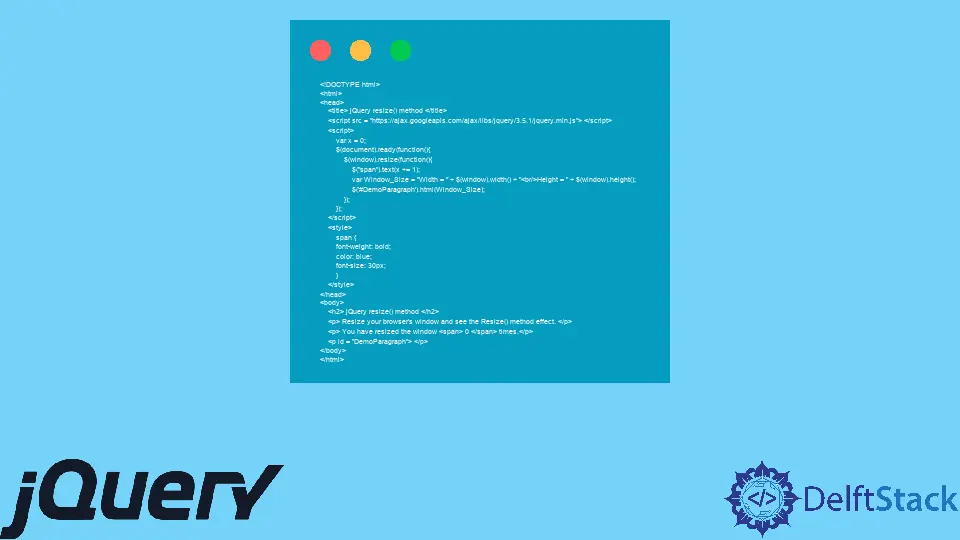
The resize()
method is a built-in function in jQuery used when the browser window changes size. This tutorial demonstrates how to use the resize()
method in jQuery.
Use the resize()
Method in jQuery
The resize()
method works similar to the onresize()
method of the JavaScript. They are used to resize the window.
The syntax for the resize()
method is:
$(selector).resize(function)
The selector
can be the window. And the function
is an optional parameter that will be called when the resize()
method is called; this function
is also called the handler.
The code in the handler should never be based on the number of times the handler is called because this event can be sent continuously until the resizing is in progress. The return value for this method is the selected elements with resized attributes.
It should also be mentioned here that the resize()
method is a jQuery version of on("resize", handler)
. So, similar to that, detaching is possible using the .off("resize")
method.
Let’s try an example:
<!DOCTYPE html>
<html>
<head>
<title> jQuery resize() method </title>
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"> </script>
<script>
var x = 0;
$(document).ready(function(){
$(window).resize(function(){
$("span").text(x += 1);
var Window_Size = "Width = " + $(window).width() + "<br/>Height = " + $(window).height();
$('#DemoParagraph').html(Window_Size);
});
});
</script>
<style>
span {
font-weight: bold;
color: blue;
font-size: 30px;
}
</style>
</head>
<body>
<h2> jQuery resize() method </h2>
<p> Resize your browser's window and see the Resize() method effect. </p>
<p> You have resized the window <span> 0 </span> times.</p>
<p id = "DemoParagraph"> </p>
</body>
</html>
The code above uses the resize()
method to show the width and height of the window on resizing. See output:
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook