jQuery replace() Method
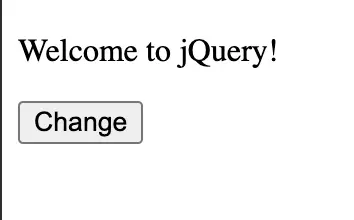
This post will discuss the jQuery replacement capability, which returns a new string after replacing every goal detail with a matched element. This approach may be used to replace the occurrence of any string in a sentence or a group of strings.
Only the primary example may be changed in the replace()
approach. The global modifier (g
) must be used to eliminate all the occurrences.
replace()
in jQuery
jQuery offers several functionalities to help manage the DOM, considered one of them being DOM replacement. Using the replace()
approach of jQuery, we will find and replace all of the occurrences of a selected substring in a string or a string in a collection of strings.
jQuery additionally offers replaceAll()
and replaceWith()
methods for DOM manipulation with replace
feature.
replaceAll()
method replaces each target element with a set of matched elements. replaceWith()
method replaces each element with the new content provided and returns the removed elements set.
Syntax:
string.replace (/[oldString]/+/g, 'newString')
$(content).replaceAll(selector)
$(selector).replaceWith(content, function(i))
- The
content
is a required parameter that specifies the content to be inserted, possible values being HTML elements, jQuery objects, and HTML elements. - The
function(i)
is an optional parameter that specifies a function that returns the content to be replaced.
Let’s understand it with the following simple example.
<p>Welcome to jQuery!</p>
<button >
Change
</button>
$(document).ready(function() {
$('button').on('click', () => {
$('p').text((index, text) => {
return text.replace(/jQuery/g, 'Delftstack');
});
})
});
In the above example, the jQuery replace()
method searches for the specified string value jQuery
and returns the specified replace
value Delftstack
.
Try and run the above code snippet in any browser that supports jQuery, which will display the result.
Before replace()
:
After replace()
:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn