How to Refresh the Page in jQuery
-
Use the
location.reload()
Method to Refresh the Page in jQuery -
Use the
history.go()
Method to Refresh the Page in jQuery
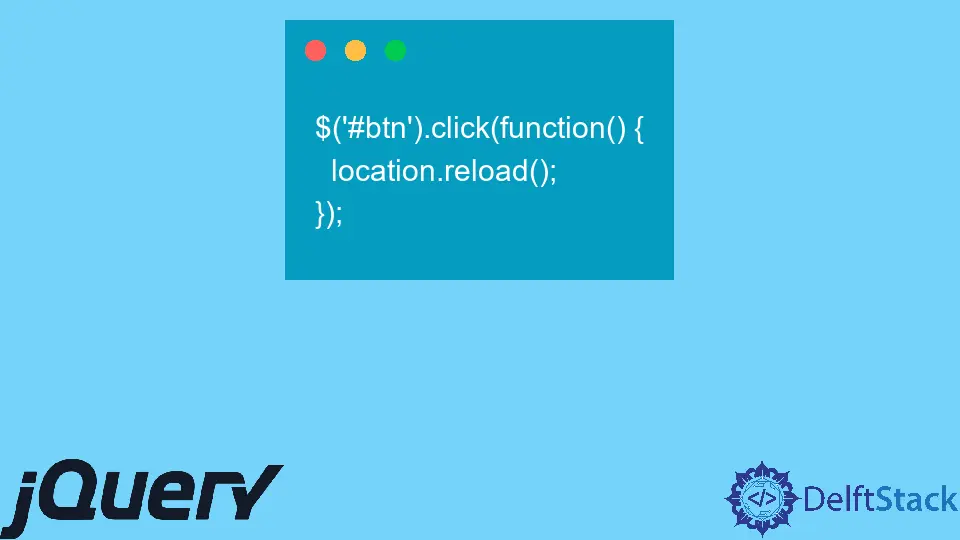
In today’s post, we’ll learn how to refresh the page using location
and history
objects in jQuery.
Use the location.reload()
Method to Refresh the Page in jQuery
Uniform Resource Locator is the page location, represented by the location
interface. Changes made to it are reflected in the object to which it refers.
The document and window interfaces have an associated location that can be accessed via Document.location
and Window.location
, respectively.
Like the Refresh button, the location.reload()
technique reloads the existing URL. location.reload()
is a well-known JavaScript method; hence, you don’t need jQuery for it.
Reloading may hang and throw a SECURITY_ERROR
DOMException. This takes place if the foundation of the script calls location.reload()
differs from the origin of the page that owns the location
object.
Syntax:
reload();
Let’s understand it with the following example.
HTML Code:
<p>Hello World!</p>
<button type="button" id="btn">Reload</button>
JavaScript Code:
$('#btn').click(function() {
location.reload();
});
Attempt to run the above code snippet in any browser that supports jQuery. It’s going to reload the page.
Use the history.go()
Method to Refresh the Page in jQuery
The history.go()
method loads a specific page of session history. You can use it to move back and forth in history based on the value of a parameter; this method is asynchronous.
The history.go()
method loads a URL from the browser’s history based on the parameter passed. If the given parameter is 0
, it will reload the current page.
Syntax:
history.go(0);
Let’s understand it with the following example.
HTML Code:
<p>Hello World!</p>
<button type="button" id="btn">Reload</button>
JavaScript Code:
$('#btn').click(function() {
history.go(0);
});
Attempt to run the above code snippet in any browser that supports jQuery. It’s going to reload the page.
View the demo here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn