How to Use Hover in jQuery
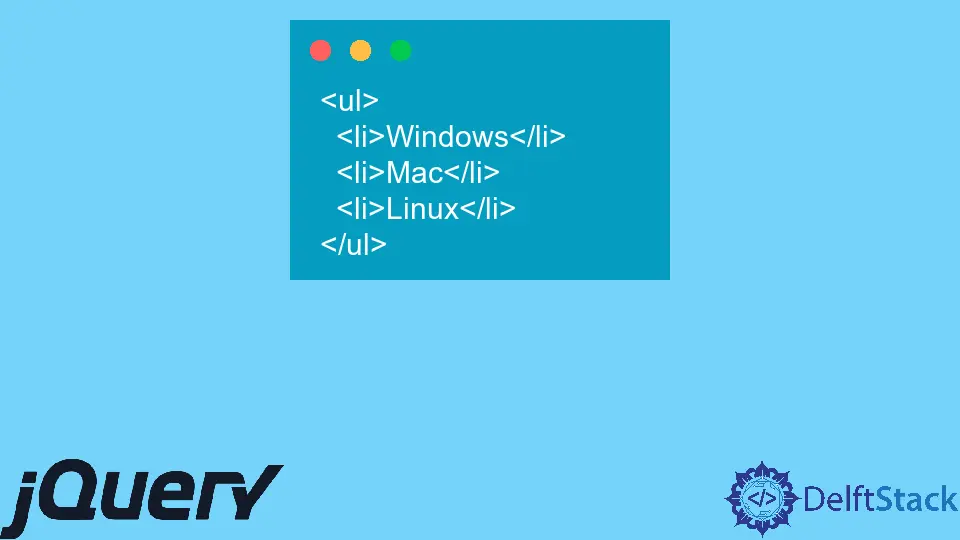
In today’s post, we will learn about the .hover()
event in jQuery.
Use .hover()
in jQuery
JQuery’s .hover()
approach binds one or both handlers to the match factors to complete simultaneously as the mouse cursor enters and exits the elements. Depending on the binder handlers, there are three varieties of parameters that the .hover()
approach can take.
Syntax:
.hover(handlerInFn, handlerOutFn).hover(handlerInOutFn)
Parameters:
handlerInFn
: When the mouse pointer enters the element, this function needs to be called/executed.handlerOutFn
: When the mouse pointer leaves the element, this function needs to be called/executed.handlerInOutFn
: When the mouse pointer enters or leaves the element, this function needs to be called/executed.
The .hover()
approach binds controllers for a mouse to move in and out of activities. You can use it to observe the element’s behavior at a given time when the mouse is inside the detail.
The .hover()
technique, even if you pass a single function, runs that handler on both mouse input and output. This shall we the person use jQuery’s various toggle techniques inside the handler or respond extraordinarily within the handler depending on the event.type
.
You can find more information in the documentation about the .hover()
approach here.
Let’s understand .hover()
with the following example:
HTML code:
<ul>
<li>Windows</li>
<li>Mac</li>
<li>Linux</li>
</ul>
JavaScript code:
$('li').hover(function() {
console.log(this.textContent)
});
Using the ul
element, we have described an operating system list within the above example. When you start hovering over the listing, it will follow the hovering impact to the element, and the appropriate function is then executed.
Within the above instance, when you begin hovering over Linux
, it will print the text value for the element.
Try to run the above code snippet in any browser that supports jQuery. It will show the result given below:
Output:
"Linux"
See the working demo of the code here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn