How to Handle Button Click Event in jQuery
- Understanding jQuery and Button Click Events
-
Using the
.click()
Method -
Using the
.on()
Method for Event Delegation -
Triggering Events with
.trigger()
- Conclusion
- FAQ
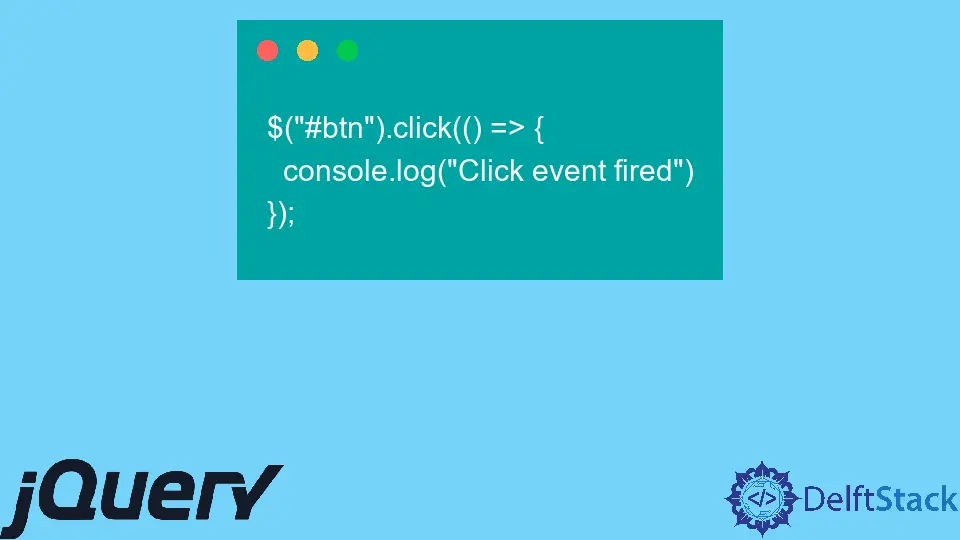
In today’s post, we’ll learn about handling button click events in jQuery. This popular JavaScript library simplifies HTML document traversing, event handling, and animation, making it easier for developers to create dynamic web applications. The button click event is a fundamental aspect of user interaction on the web. Whether you want to trigger a function, change the content of a webpage, or perform an AJAX call, handling button clicks effectively is essential. By the end of this article, you will have a solid understanding of how to manage button click events using jQuery, along with practical examples to illustrate each method. Let’s dive in!
Understanding jQuery and Button Click Events
Before we jump into the code, it’s important to understand what jQuery is and how it interacts with button click events. jQuery is a fast, small, and feature-rich JavaScript library that makes things like HTML document traversal and manipulation, event handling, and animation much simpler.
Button click events allow you to respond to user interactions. When a user clicks a button, you can execute a specific function or series of functions. This is crucial for creating interactive web applications. jQuery provides several methods to handle these events, including .click()
, .on()
, and .trigger()
.
Let’s explore these methods in detail.
Using the .click()
Method
One of the simplest ways to handle button click events in jQuery is by using the .click()
method. This method attaches an event handler function to the specified element.
Here’s how you can use it:
$(document).ready(function() {
$("#myButton").click(function() {
alert("Button was clicked!");
});
});
In this example, we wait for the document to be fully loaded using $(document).ready()
. Then, we select the button with the ID myButton
and attach a click event handler. When the button is clicked, an alert box will pop up with the message “Button was clicked!”
This method is straightforward and easy to implement, making it an excellent choice for beginners. However, it’s worth noting that .click()
is a shorthand method for .on('click', ...)
, which we will discuss next.
Output:
The .click()
method is great for simple interactions. However, if you need to manage multiple events or want to delegate events for dynamically added elements, the .on()
method is more appropriate.
Using the .on()
Method for Event Delegation
The .on()
method in jQuery is more versatile than .click()
. It allows you to attach event handlers to elements, including those that are added to the DOM after the initial page load. This is particularly useful for applications that dynamically add content.
Here’s an example of using .on()
:
$(document).ready(function() {
$("#parentDiv").on("click", ".childButton", function() {
alert("Child button clicked!");
});
});
In this case, we attach a click event to #parentDiv
, which listens for clicks on any buttons with the class .childButton
. This means that even if new buttons are added to #parentDiv
after the page loads, the event handler will still work.
Using the .on()
method not only enhances performance by reducing the number of event handlers but also provides greater flexibility. This method is especially beneficial in applications where elements are frequently added or removed.
Triggering Events with .trigger()
Sometimes, you may want to programmatically trigger a button click event. This can be done using the .trigger()
method. This method simulates a click event on the selected element, allowing you to execute the associated event handler.
Here’s how you can use it:
$(document).ready(function() {
$("#myButton").click(function() {
alert("Button was clicked!");
});
$("#triggerButton").click(function() {
$("#myButton").trigger("click");
});
});
In this example, clicking #triggerButton
will programmatically trigger the click event on #myButton
. Thus, the alert will appear just as if the user had clicked #myButton
directly.
The .trigger()
method can be particularly useful in scenarios where you want to initiate a sequence of events or create complex interactions without requiring the user to click on the element directly.
Conclusion
Handling button click events in jQuery is an essential skill for any web developer. Whether you choose the .click()
, .on()
, or .trigger()
method, each has its strengths and use cases. By understanding these methods, you can create more interactive and engaging web applications. Remember to consider the context of your application when choosing which method to use. With practice, you’ll find that managing button click events becomes second nature.
FAQ
-
What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal, event handling, and animation. -
How do I attach a click event to a button?
You can use the.click()
method or the.on()
method in jQuery to attach a click event to a button. -
What is the difference between .click() and .on()?
The.click()
method is a shorthand for attaching click events, while.on()
is more versatile and can handle events for dynamically added elements. -
Can I trigger a button click programmatically?
Yes, you can use the.trigger()
method to programmatically trigger a click event on a button. -
What is event delegation in jQuery?
Event delegation is a technique where you attach an event handler to a parent element, allowing it to manage events for child elements, even if they are added dynamically.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn