jQuery Multiple Selectors
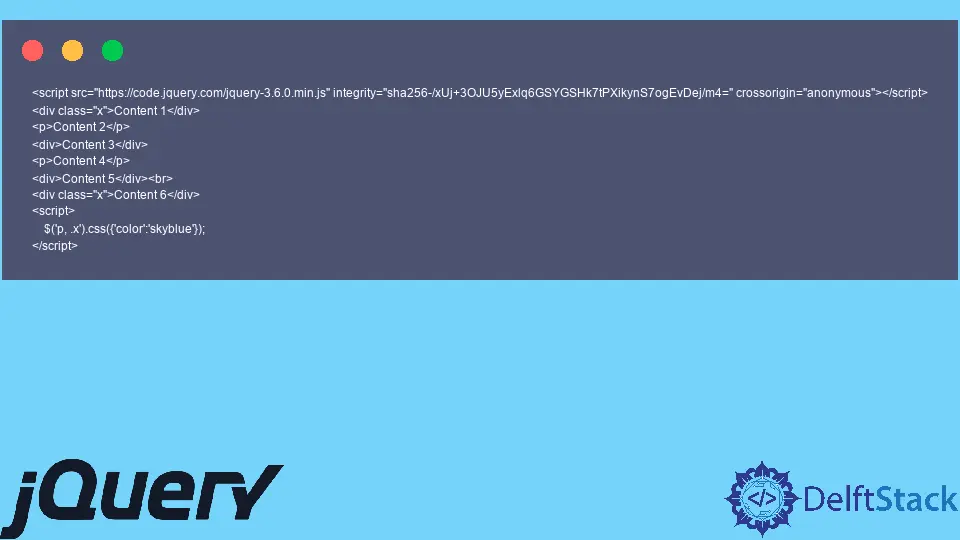
jQuery, the library that ensures few lines of code for greater solutions, technically works under the heed in pretty much complex and fancy ways. Consequently, the performance cannot always be measured too explicitly.
However, when working with multiple selectors, one might have queries regarding performance with the general implementation. It does not vary much, according to most developers.
The following example will work with the element
selector and class
selector. The drive is effortless and significant when handling vast variants of content.
Use of Multiple Selectors in jQuery
The jQuery multiple selectors’ convention allows to grab selectors of different concerns and can modify or update according to requirement. This convention does not have any different syntax other than the basic way of initializing the selectors.
All emphasized here is to select the selectors and separate them with a comma.
Here, we will have multiple HTML elements (div
and p
), and some of the div
will be associated with a specific class x
. We aim to grab all the div
with class x
and the p
tags and identify them with a different color.
Let’s go through the code fence for the demonstration.
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script>
<div class="x">Content 1</div>
<p>Content 2</p>
<div>Content 3</div>
<p>Content 4</p>
<div>Content 5</div><br>
<div class="x">Content 6</div>
<script>
$('p, .x').css({'color':'skyblue'});
</script>
Output:
Several other conventions will give more flexibility in different aspects of development. You might visit here for a tabular detail on the existing ways of dealing with multiple selectors.
Also, the documentation defines the rest more clearly.