How to Loop Through Array in jQuery
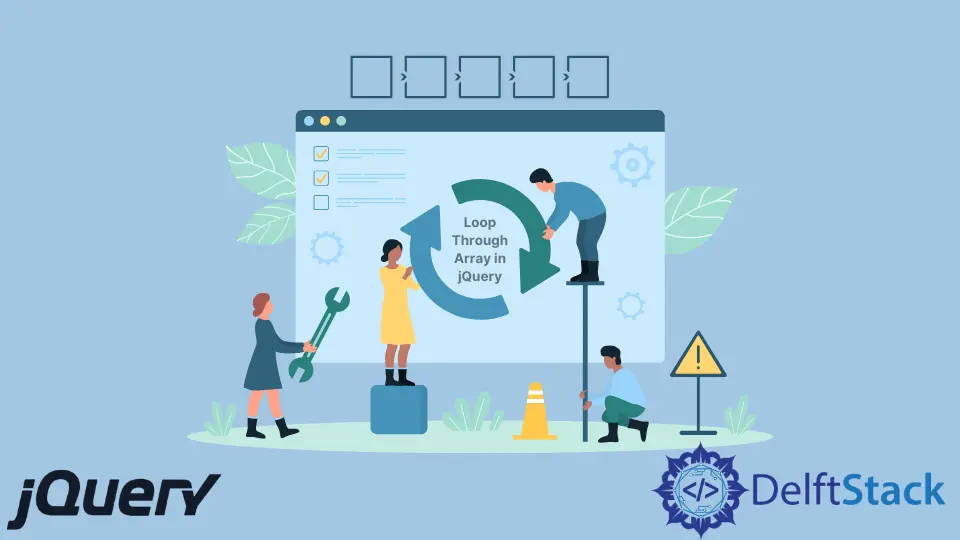
In JavaScript, we have a wide range of possibilities through which we can implement the iteration of an array or any other object. In jQuery, we have only one way to initiate an iterator: the $.each()
method.
This short article will show how to loop through an array using the $.each()
method and the for
loop in jQuery.
the $.each()
Method and for
Loop in jQuery
If we differentiate between the for
loop and the $.each()
method, then the for
loop is more explicit in showing how the operation flow is being conducted. It also makes comparative more lines of code.
On the contrary, the whole process is abstracted in the jQuery method. All it requires is the initiation of the method by $.each()
and adding a driver function having the index and value as parameters.
In the following section, we will observe how the $.each()
function and the for
loop works. We can also implement the traditional way to iterate an array instead of the defined jQuery method.
Use for
Loop in jQuery
Code Snippet:
var arr = [7, 4, 2, 4];
var sum = 0;
for (var i = 0; i < arr.length; i++) {
sum += arr[i];
}
console.log('Using for loop and sum: ' + sum);
Output:
As can be seen, there is no mention of any jQuery window function. So, we cannot say it is a dedicated jQuery way of iterating arrays.
Then again, jQuery is a library of JavaScript, so it sums up the usability.
Use the $.each()
Method to Iterate an Array in jQuery
We will take an array, and in the $.each()
method, we will integrate a function with the parameters named index and value. We will not set any variable to showcase the iteration.
Rather we can easily sum the values in one line of code.
Code Snippet:
var arr = [7, 5, 9, 4];
var sum = 0;
$.each(arr, (index, val) => {
sum += val;
});
console.log('Using jQuery each and the Sum: ' + sum);
Output: