How to Update innerHTML Using jQuery
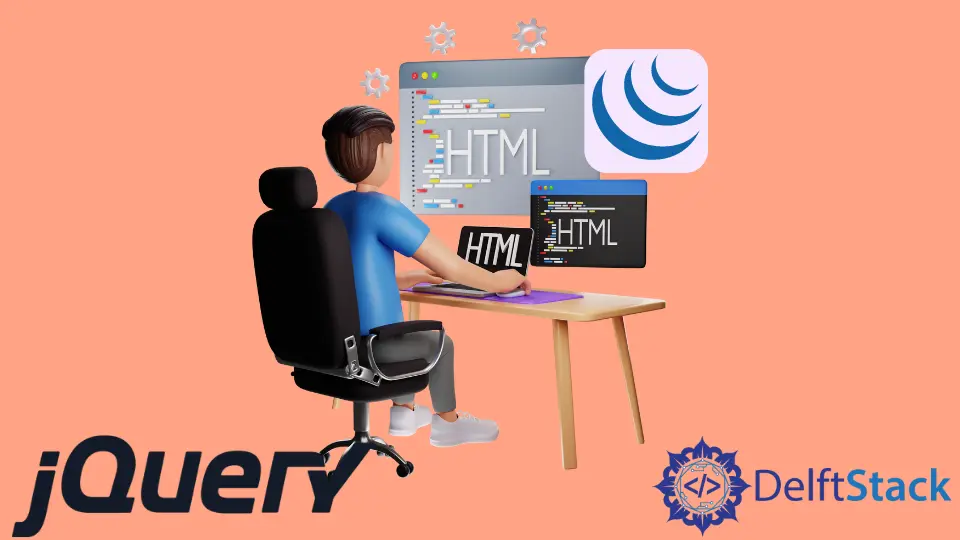
In today’s post, we’ll learn about updating or replacing the inner HTML of an element in jQuery.
Update innerHTML
Using jQuery
jQuery provides the .html()
method to set the HTML contents of each element in the set of matched elements.
Syntax:
.html(htmlString).html(function)
- The
htmlString
is an HTML string set as the content of each matching element. - This is a
function
that returns the HTML content material to the set. The old HTML value and index of the element in the set are considered arguments.
Before calling the function, jQuery empties the element. Then it uses the old HTML argument to consult the old content. this
can refer to the current element within the set inside the function.
When .html()
is used to set the content of an element, any content in that element is completely replaced with the new content. Additionally, jQuery gets rid of other constructs like information and event handlers from child elements earlier than replacing those elements with brand new content.
The innerHTML
property of the browser is used in this method.
Some browsers may not generate a DOM that exactly replicates the provided HTML source. Use the .text()
method to set the content of a script
element that does not contain HTML, rather than the .html()
method.
Let’s understand it with the following example.
HTML Code:
<div class="txt-container">Hello World! Welcome to the DelftStack.</div>
<button type="button">Change the text</button>
JavaScript Code:
$('button').click(() => {
$('div.txt-container')
.html('<p>Thank you for visiting <em>DelftStack!</em></p>');
})
In the above example, we have defined the div
element, which prints the Hello World! Welcome to the DelftStack.
message. When you click the Change the text
button, it will update the DOM with the new paragraph tag.
Try to run the above code snippet in any browser that supports jQuery. It’s going to display the following result.
Output Before Changing the Text:
Output After Changing the Text:
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn