jQuery indexof() Method
- What is the jQuery indexOf() Method?
- Using indexOf() with Strings
- Practical Applications of indexOf()
- Conclusion
- FAQ
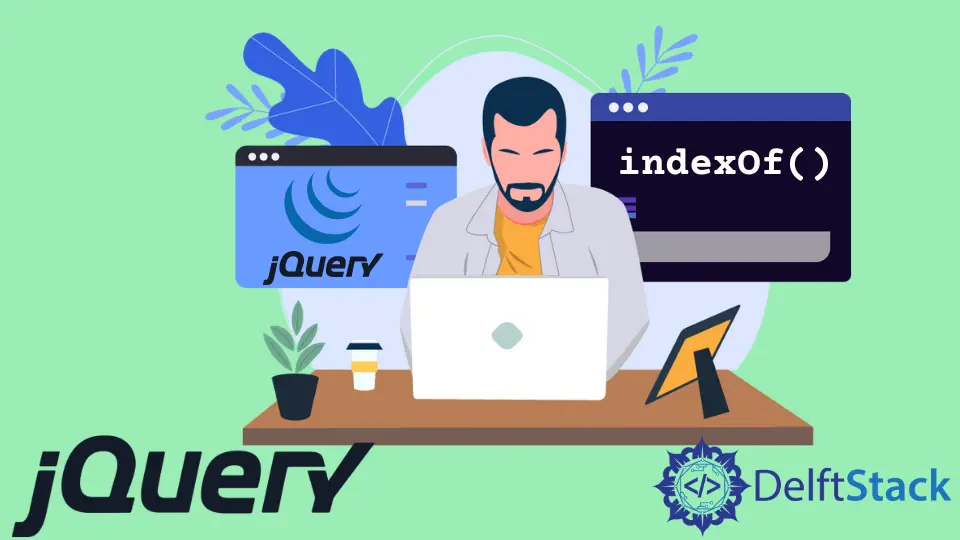
In the world of web development, jQuery remains a powerful tool for simplifying JavaScript tasks. One of its handy functions is the indexOf()
method. This method plays a crucial role in searching for specific elements within an array or string. Whether you’re a beginner or a seasoned developer, understanding how to effectively utilize the indexOf()
method can streamline your coding process and enhance your applications. In this post, we’ll dive deep into the jQuery indexOf()
method, explore its syntax, and illustrate its practical applications with examples. By the end, you’ll be well-equipped to leverage this method in your projects.
What is the jQuery indexOf() Method?
The indexOf()
method in jQuery is used to determine the position of a specified value within an array or string. If the value is found, it returns the index of the first occurrence; otherwise, it returns -1. This method is particularly useful when you need to check if an element exists within a collection or to find its position for further manipulation.
Syntax
The basic syntax for the indexOf()
method is as follows:
array.indexOf(searchElement, fromIndex)
searchElement
: The value to search for within the array.fromIndex
(optional): The index at which to begin the search. Defaults to 0.
Example of indexOf() with Arrays
Let’s look at a practical example that demonstrates how to use the indexOf()
method with an array.
var fruits = ["apple", "banana", "orange", "mango"];
var position = fruits.indexOf("orange");
console.log(position);
Output:
2
In this example, we have an array of fruits. By calling indexOf()
with “orange” as the search element, we receive 2
as the output, indicating that “orange” is located at index 2 of the array.
The indexOf()
method is straightforward and efficient, making it easy to locate elements within an array. If the element is not found, the method returns -1, which can be useful for conditional checks in your code.
Using indexOf() with Strings
The indexOf()
method is not limited to arrays; it can also be applied to strings. This allows developers to find the position of a substring within a larger string.
Example of indexOf() with Strings
Here’s an example of how to use indexOf()
with strings:
var text = "Hello, welcome to the world of jQuery.";
var position = text.indexOf("welcome");
console.log(position);
Output:
7
In this case, the indexOf()
method searches for the substring “welcome” within the string text
. The output 7
indicates that “welcome” starts at index 7. If you were to search for a substring that doesn’t exist in the string, like “Python,” the method would return -1.
Using indexOf()
with strings can be particularly useful for tasks like validating input, searching for keywords, or parsing text data. The method is case-sensitive, so “welcome” and “Welcome” would yield different results.
Practical Applications of indexOf()
Understanding the practical applications of the indexOf()
method can help you implement it effectively in your projects. Here are some scenarios where indexOf()
can be particularly beneficial:
-
Element Existence Check: Before performing operations on an element, you can check if it exists in an array or string. This prevents errors and enhances the robustness of your code.
-
Dynamic Content Manipulation: In interactive applications, you might need to manipulate content based on user input. The
indexOf()
method can help locate elements dynamically, allowing for real-time updates of your UI. -
Data Validation: When working with user input, you can use
indexOf()
to validate whether certain keywords or values are present, ensuring that the data meets your application’s requirements. -
Search Functionality: Implementing search features in your application becomes easier with
indexOf()
. You can quickly locate items in arrays or strings, enhancing the user experience.
Example of Practical Application
Here’s a practical example that combines several concepts:
var colors = ["red", "blue", "green", "yellow"];
var userColor = "green";
if (colors.indexOf(userColor) !== -1) {
console.log(userColor + " is available in the color list.");
} else {
console.log(userColor + " is not available.");
}
Output:
green is available in the color list.
In this example, we check if the user’s selected color exists in the colors
array. If it does, we inform the user; otherwise, we let them know it’s not available. This pattern is common in user interfaces where options are dynamically validated against a list.
Conclusion
The jQuery indexOf()
method is a versatile and powerful tool for developers. Its ability to quickly find the position of elements in arrays and strings makes it invaluable for various applications, from data validation to dynamic content manipulation. By mastering the indexOf()
method, you can streamline your coding process and enhance the functionality of your web applications. Whether you’re building a simple webpage or a complex application, integrating indexOf()
into your toolkit will undoubtedly prove beneficial.
FAQ
-
What does the jQuery indexOf() method do?
The jQueryindexOf()
method returns the first index of a specified value in an array or string, or -1 if it is not found. -
Is indexOf() case-sensitive?
Yes, theindexOf()
method is case-sensitive, meaning that it distinguishes between uppercase and lowercase letters. -
Can I use indexOf() on objects?
No, theindexOf()
method is designed for use with arrays and strings. It does not work with objects. -
What happens if the element is not found?
If the specified element is not found, theindexOf()
method returns -1. -
Can I specify where to start the search with indexOf()?
Yes, you can specify the starting index by passing a second argument to theindexOf()
method.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn