How to Get URL Parameter in jQuery
- Understanding URL Parameters
- Method 1: Using jQuery to Get URL Parameters
- Method 2: Parsing the URL Manually with jQuery
- Method 3: Using jQuery Plugins for Enhanced Functionality
- Conclusion
- FAQ
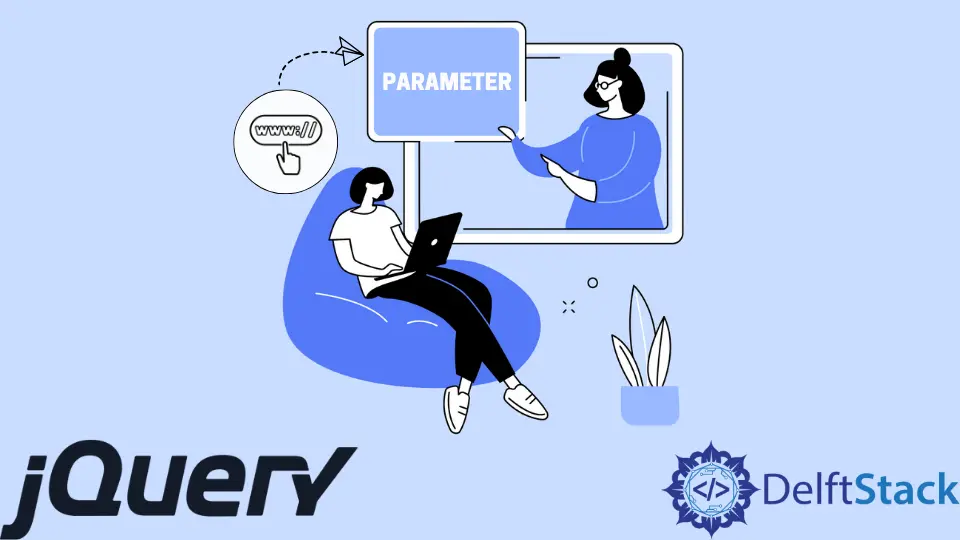
In today’s digital landscape, understanding how to work with URL parameters is essential for web developers. URL parameters allow you to pass data between pages, making your web applications dynamic and interactive. If you’ve ever wanted to retrieve specific values from a URL in your jQuery projects, you’re in the right place.
This article will guide you through the process of getting URL parameters using jQuery. We’ll cover several methods, providing clear examples and explanations. By the end of this post, you’ll have a solid grasp of how to manipulate URL parameters effectively. So, let’s dive in!
Understanding URL Parameters
Before we jump into the code, let’s clarify what URL parameters are. URL parameters are key-value pairs added to the end of a URL, usually following a question mark. For example, in the URL https://example.com/page?name=John&age=30
, name
and age
are parameters. The values John
and 30
are associated with these keys, respectively. This mechanism is commonly used to send data to web applications, such as user input from forms or filter settings.
Method 1: Using jQuery to Get URL Parameters
One of the simplest ways to retrieve URL parameters in jQuery is by using the window.location.search
property. This property returns the query string part of the URL, which you can then parse to extract the parameters you need.
Here’s how you can achieve this:
function getUrlParameter(name) {
const urlParams = new URLSearchParams(window.location.search);
return urlParams.get(name);
}
// Example usage
const name = getUrlParameter('name');
console.log(name);
Output:
John
In this code snippet, we define a function called getUrlParameter
that takes a parameter name as an argument. It uses the URLSearchParams
interface to parse the query string. The get
method retrieves the value associated with the specified parameter. In the example usage, we call the function with the parameter name
, and it returns John
, which we then log to the console.
This method is straightforward and leverages modern JavaScript features, making it efficient for retrieving URL parameters. It works in most modern browsers and is particularly useful for quick implementations.
Method 2: Parsing the URL Manually with jQuery
If you prefer to avoid using the URLSearchParams
interface, you can manually parse the URL parameters. This method involves splitting the query string and iterating through the key-value pairs.
Here’s how to do it:
function getUrlParameter(name) {
const queryString = window.location.search.substring(1);
const params = queryString.split("&");
for (let i = 0; i < params.length; i++) {
const pair = params[i].split("=");
if (decodeURIComponent(pair[0]) === name) {
return decodeURIComponent(pair[1] || '');
}
}
return null;
}
// Example usage
const age = getUrlParameter('age');
console.log(age);
Output:
30
In this example, we extract the query string from window.location.search
and remove the leading question mark using substring(1)
. We then split the string into an array of parameters using split("&")
. Next, we loop through each parameter, splitting it into key and value pairs. If the key matches the parameter name we are looking for, we return the decoded value. If the parameter is not found, we return null
.
This approach is more manual but offers a deeper understanding of how URL parameters are structured. It can be useful in scenarios where you want to customize the parsing logic or handle specific cases.
Method 3: Using jQuery Plugins for Enhanced Functionality
If you’re working on a larger project or need more advanced features, consider using a jQuery plugin designed for URL parameter handling. One popular option is the jQuery BBQ plugin, which provides a more robust way to manage URL parameters and hash fragments.
Here’s how you can use jQuery BBQ:
// Assuming jQuery and jQuery BBQ are included
$(document).ready(function() {
const name = $.bbq.getState('name');
console.log(name);
});
// Example usage in URL: https://example.com/page#name=John
Output:
John
In this code snippet, we first ensure that jQuery and the jQuery BBQ plugin are included in our project. The $.bbq.getState
function retrieves the value of the name
parameter from the URL hash. This method is particularly useful for single-page applications (SPAs) where you want to manage state using URL hashes.
Using a plugin like jQuery BBQ simplifies the process of handling URL parameters and provides additional features, such as automatic updates to the URL when state changes. This can enhance the user experience and make your application feel more dynamic.
Conclusion
Retrieving URL parameters in jQuery is a fundamental skill for any web developer. Whether you choose to use built-in JavaScript methods, manually parse the parameters, or leverage a plugin, understanding how to manipulate URL parameters can significantly enhance your web applications. With the techniques discussed in this article, you can easily access and utilize URL parameters, making your projects more interactive and user-friendly. Happy coding!
FAQ
-
How do I get the full URL in jQuery?
You can usewindow.location.href
to get the full URL of the current page. -
Can I retrieve multiple URL parameters at once?
Yes, you can call your parameter retrieval function multiple times for different parameters. -
Is it necessary to use jQuery to get URL parameters?
No, you can use plain JavaScript to achieve the same results without jQuery. -
What if a URL parameter contains special characters?
You should usedecodeURIComponent
to properly decode the parameter values. -
Are there any performance concerns when using URL parameters?
Generally, accessing URL parameters is efficient, but excessive use of complex parsing logic may slow down performance.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn