How to Get Data Attribute Values in jQuery
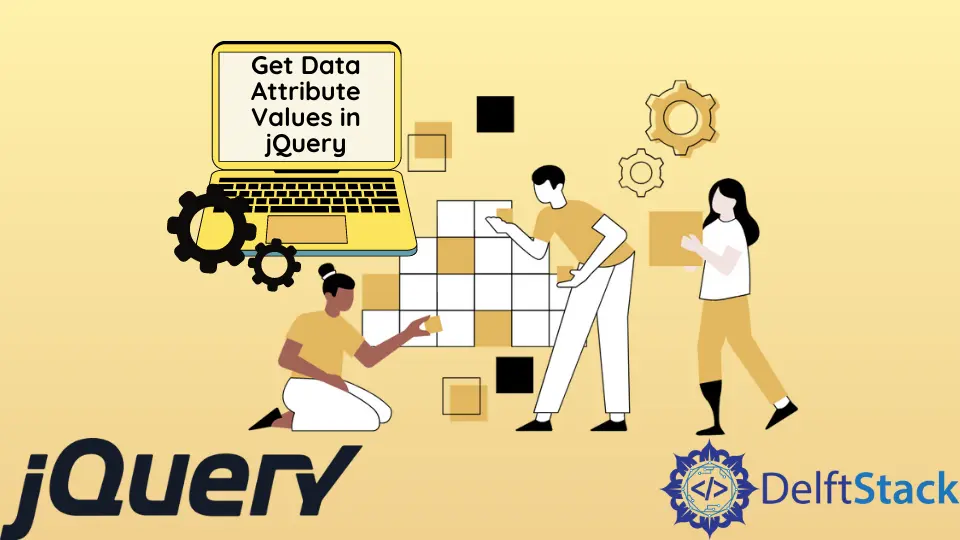
jQuery provides two ways to get the data attribute values: the first is the .data()
method, and the second is the .attr()
method. Today’s post will teach you about getting the data attribute values in jQuery.
Use .data()
and .attr()
to Get Data Attribute Values in jQuery
Data set by data()
or by an HTML5 data-*
attribute will be returned by the .data()
method. This method returns arbitrary data associated with the first element in the jQuery collection.
Without passing the parameter, .data()
returns a JavaScript object. This JavaScript object contains each saved value as a resource or an asset.
The object may be used immediately to get the record values.
Syntax:
.data(key)
This method accepts the name of the data stored as a key. You can find more information about the .data()
method in the documentation for .data()
.
We can also get the value of a characteristic for the first detail in the set of matched factors. The .attr()
approach receives the characteristic value for best the primary element in the matched set.
To get the value for every detail, we can use a looping construct inclusive of jQuery’s .each()
or .map()
method.
Syntax:
.attr(attributeName)
This method accepts the attribute name to get the data associated with the attribute. Using the jQuery .attr()
method to obtain the value of an element’s characteristic has the following main advantages.
Without delay, it can be called on a jQuery object and chained to different jQuery methods. The values of some attributes may be stated unevenly throughout browsers and even throughout versions of a single browser; the .attr()
approach reduces such inconsistencies.
You can find more information about the .attr()
method in the documentation for .attr()
.
Let’s understand it with the following example.
Example code:
<button data-id="btn-1" value="Hello">Hello World!</button>
console.log($('button').attr('data-id'));
console.log($('button').data('id'));
We have described a button with a data-id
attribute set to btn-1
and a value of Hello
within the above example. Using the data()
or attr()
method, you can access the data attribute.
Attempt to run the above code snippet in any browser that supports jQuery. It is going to display the result below.
Output:
"btn-1"
"btn-1"
You may run the above example code here.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn